yqt5 根据坐标点绘图,坐标图中会有10个点相连接,且其中某两个点连接线段是一段圆弧,图像绘制好后将其置于窗口中心,且图像大小适应窗口大小
时间: 2024-04-30 18:23:32 浏览: 11
以下是一个绘制带圆弧的坐标图的示例代码,使用 PyQt5 库。在代码中,我们定义了一个类 `CoordinateWidget`,它继承自 QWidget,用于绘制坐标图。在 `paintEvent` 方法中,我们使用 QPainter 对象绘制了一个带圆弧的多边形,其中圆弧部分使用 `QPainterPath` 对象实现。
为了将绘制好的坐标图置于窗口中心并适应窗口大小,我们在 `resizeEvent` 方法中重新计算了绘制坐标图的矩形区域,并使用 `QPainter.translate` 和 `QPainter.scale` 方法对绘制坐标图的坐标系进行平移和缩放。
完整代码如下:
```
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QPainter, QPainterPath
from PyQt5.QtCore import Qt, QRectF
class CoordinateWidget(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.points = [
(50, 50),
(100, 150),
(150, 150),
(200, 50),
(250, 100),
(200, 150),
(150, 100),
(100, 50),
(75, 100),
(125, 125)
]
def paintEvent(self, event):
qp = QPainter(self)
qp.setRenderHint(QPainter.Antialiasing)
path = QPainterPath()
path.moveTo(*self.points[0])
for i in range(1, len(self.points)):
if i == 1:
path.lineTo(*self.points[i])
elif i == 9:
path.arcTo(QRectF(self.points[i][0]-25, self.points[i][1]-25, 50, 50), 45*16, 180*16)
else:
path.lineTo(*self.points[i])
path.closeSubpath()
qp.drawPath(path)
def resizeEvent(self, event):
rect = self.geometry()
size = min(rect.width(), rect.height())
x = rect.x() + (rect.width() - size) // 2
y = rect.y() + (rect.height() - size) // 2
self.setContentsMargins(x, y, 0, 0)
qp = QPainter(self)
qp.translate(size/2, size/2)
qp.scale(size/300, size/300)
self.update()
if __name__ == '__main__':
app = QApplication(sys.argv)
widget = CoordinateWidget()
widget.resize(500, 500)
widget.show()
sys.exit(app.exec_())
```
在代码中,我们将坐标点保存在 `points` 列表中。在 `paintEvent` 方法中,我们使用 `QPainterPath` 对象绘制了一个多边形,其中使用 `arcTo` 方法绘制了圆弧部分。在 `resizeEvent` 方法中,我们重新计算了绘制坐标图的矩形区域,并使用 `QPainter.translate` 和 `QPainter.scale` 方法对绘制坐标图的坐标系进行平移和缩放,使其适应窗口大小。
相关推荐
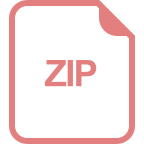
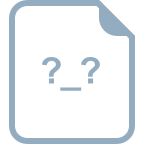
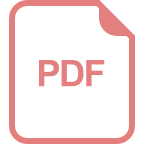





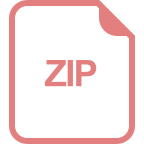
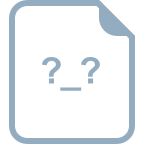
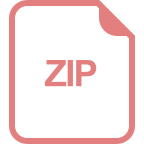
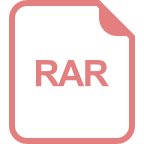
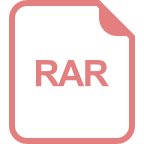