createprocess传int型参数
时间: 2023-12-30 22:02:28 浏览: 148
CreateProcess函数是Windows API的一个函数,用于创建新的进程和线程。如果要传递int型参数,则可以使用命令行参数的方式。
例如,假设我们要创建一个进程,该进程需要接收一个int类型的参数num,可以这样做:
1. 将参数num转换成字符串。可以使用itoa函数将其转换为字符串形式。
2. 将该字符串作为命令行参数传递给CreateProcess函数。
下面是一个示例代码:
```
#include <stdio.h>
#include <windows.h>
int main()
{
int num = 123;
char arg[16];
itoa(num, arg, 10);
char cmdLine[32] = "child.exe ";
strcat(cmdLine, arg);
STARTUPINFO si = {0};
si.cb = sizeof(si);
PROCESS_INFORMATION pi = {0};
if (CreateProcess(NULL, cmdLine, NULL, NULL, FALSE, 0, NULL, NULL, &si, &pi))
{
printf("Child process created.\n");
WaitForSingleObject(pi.hProcess, INFINITE);
CloseHandle(pi.hProcess);
CloseHandle(pi.hThread);
}
else
{
printf("CreateProcess failed.\n");
}
return 0;
}
```
在上述示例代码中,我们创建了一个名为child.exe的子进程,并将参数num作为命令行参数传递给该进程。在子进程中,可以使用GetCommandLine函数获取命令行参数,并使用atoi函数将其转换为int类型。
下面是child.exe的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num = atoi(GetCommandLine() + 9);
printf("Received parameter: %d\n", num);
return 0;
}
```
在上述示例代码中,我们使用GetCommandLine函数获取命令行参数,并使用atoi函数将其转换为int类型。最后输出接收到的参数num。
阅读全文
相关推荐
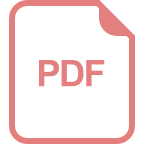
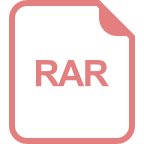

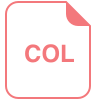
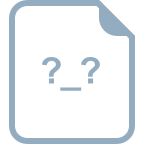
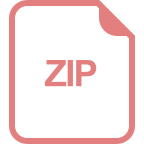
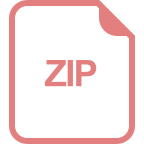
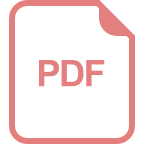
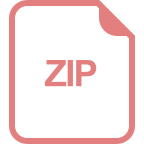
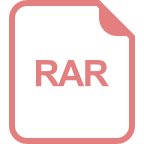
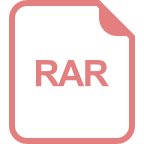
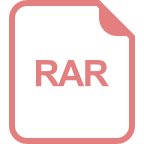