设计一People类,包含name(String)、age(int)属性与相关设定、读取方法。 设计Student类,继承People类,属性有修习的课程分数,属性名称为chinese、english、math三科目成绩,并写出设定与读取分数的方法,以及计算三科平均分数的方法。
时间: 2024-03-01 22:49:41 浏览: 199
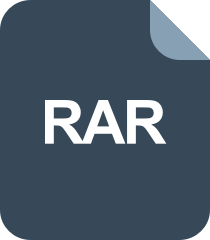
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

以下是 Java 代码实现 People 类和 Student 类:
```java
public class People {
private String name;
private int age;
public People(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
}
public class Student extends People {
private int chinese;
private int english;
private int math;
public Student(String name, int age, int chinese, int english, int math) {
super(name, age);
this.chinese = chinese;
this.english = english;
this.math = math;
}
public int getChinese() {
return chinese;
}
public int getEnglish() {
return english;
}
public int getMath() {
return math;
}
public void setChinese(int chinese) {
this.chinese = chinese;
}
public void setEnglish(int english) {
this.english = english;
}
public void setMath(int math) {
this.math = math;
}
public double getAverageScore() {
return (double) (chinese + english + math) / 3;
}
}
```
在上述代码中,People 类包含了 name 和 age 两个属性和相关的设定、读取方法,Student 类继承了 People 类,并新增了 chinese、english、math 三个属性和相关的设定、读取方法,以及计算三科平均分数的方法 getAverageScore()。
可以使用以下代码进行测试:
```java
public class Main {
public static void main(String[] args) {
Student student = new Student("张三", 18, 80, 90, 85);
System.out.println("学生姓名:" + student.getName());
System.out.println("学生年龄:" + student.getAge());
System.out.println("语文成绩:" + student.getChinese());
System.out.println("英语成绩:" + student.getEnglish());
System.out.println("数学成绩:" + student.getMath());
System.out.println("三科平均分数:" + student.getAverageScore());
}
}
```
运行后,输出结果如下:
```
学生姓名:张三
学生年龄:18
语文成绩:80
英语成绩:90
数学成绩:85
三科平均分数:85.0
```
阅读全文
相关推荐
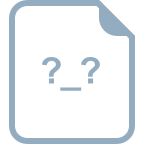
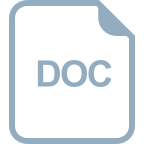


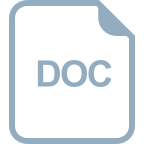
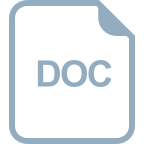
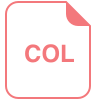
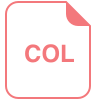
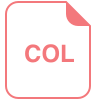
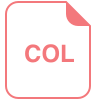
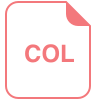

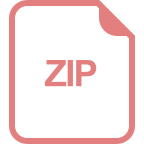