c# 查找数组中的某个元素
时间: 2023-06-24 17:03:46 浏览: 65
在 C# 中,可以使用 Array 类的 IndexOf 方法来查找数组中的某个元素。该方法返回指定元素在数组中第一次出现的索引,如果该元素不存在于数组中,则返回 -1。
以下是一个示例代码:
```csharp
int[] myArray = { 1, 3, 5, 7, 9 };
int searchValue = 5;
int index = Array.IndexOf(myArray, searchValue);
if (index != -1)
{
Console.WriteLine($"元素 {searchValue} 在数组中的索引为 {index}");
}
else
{
Console.WriteLine($"元素 {searchValue} 不存在于数组中");
}
```
输出结果为:
```
元素 5 在数组中的索引为 2
```
相关问题
C#删除数组中的某个元素
可以使用C#中的Array类的Copy方法和RemoveAt方法来删除数组中的某个元素。具体步骤如下:
1.使用Copy方法将原数组复制到一个新数组中。
2.使用RemoveAt方法删除新数组中指定位置的元素。
3.将新数组复制回原数组中。
下面是示例代码:
```csharp
int[] arr = { 1, 2, 3, 4, 5 };
int index = 2; //要删除的元素的索引
int[] newArr = new int[arr.Length - 1];
Array.Copy(arr, 0, newArr, 0, index);
Array.Copy(arr, index + 1, newArr, index, arr.Length - index - 1);
arr = newArr;
```
C# 数组中查找是否含有某个元素
在 C# 中,可以使用 `Array.IndexOf` 方法来查找一个数组中是否包含某个元素,并且返回该元素在数组中的索引位置。如果数组中不包含该元素,则返回 -1。具体用法可以参考下面的示例代码:
```csharp
using System;
class Example
{
static void Main()
{
// 定义一个包含一些字符串的数组
string[] strings = { "apple", "banana", "orange", "pear" };
// 查找数组中是否包含字符串 "orange",并返回其索引位置
int index = Array.IndexOf(strings, "orange");
if (index != -1)
{
Console.WriteLine("数组中包含字符串 'orange',索引位置为 " + index);
}
else
{
Console.WriteLine("数组中不包含字符串 'orange'");
}
}
}
```
运行上面的示例代码,如果数组中包含字符串 "orange",则会输出它在数组中的索引位置;否则会输出 "数组中不包含字符串 'orange'"。
相关推荐
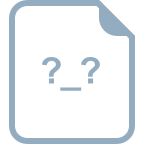
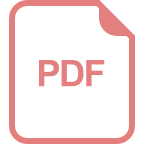












