从mysql数据库中读取数据_并保存到数组_从数据库中读取数据并存储在数组列表对象中...
时间: 2024-02-25 16:55:14 浏览: 24
好的,我可以帮你解决这个问题。
首先,你需要连接到 MySQL 数据库。这可以通过使用 Java 中的 JDBC API 来完成。以下是一个简单的连接到 MySQL 数据库的示例代码:
```java
import java.sql.*;
public class MySQLConnection {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "myusername";
String password = "mypassword";
try (Connection conn = DriverManager.getConnection(jdbcUrl, username, password)) {
System.out.println("Connected to database!");
} catch (SQLException e) {
System.err.println("Failed to connect to database");
e.printStackTrace();
}
}
}
```
在这个示例中,你需要将 `jdbcUrl`、`username` 和 `password` 替换为你自己的数据库连接信息。
一旦你连接成功,你可以使用 `Statement` 或 `PreparedStatement` 对象执行 SQL 查询并将结果存储在数组列表对象中。下面是一个简单的示例代码:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class MySQLQuery {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "myusername";
String password = "mypassword";
try (Connection conn = DriverManager.getConnection(jdbcUrl, username, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable")) {
List<String> data = new ArrayList<>();
while (rs.next()) {
String value = rs.getString("column_name");
data.add(value);
}
System.out.println("Data retrieved from database:");
System.out.println(data);
} catch (SQLException e) {
System.err.println("Failed to execute query");
e.printStackTrace();
}
}
}
```
在这个示例中,你需要将 `jdbcUrl`、`username` 和 `password` 替换为你自己的数据库连接信息,将 `mytable` 替换为你想要从中检索数据的表名,将 `column_name` 替换为你想要检索的列名。
当你运行这个示例代码时,它将从数据库中检索数据并将其存储在一个字符串列表中。你可以按照你的需要修改代码,以适应你的具体情况。
相关推荐
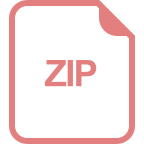
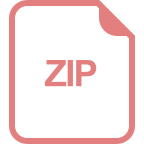
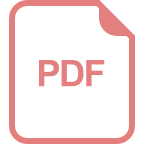
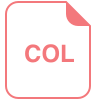
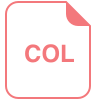
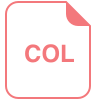
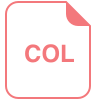
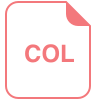









