使用hadoop编写数据生成器生成1G~10G大小的数据,字段必须包括id,日期,手机号码、型号、操作系统字段。 需要将手机号码4~9为掩码处理。
时间: 2024-03-05 15:48:53 浏览: 20
好的,我可以为您提供一个使用 Hadoop MapReduce 编写的数据生成器代码,可以生成指定大小的数据,并且包括id、日期、手机号码、型号和操作系统字段。手机号码的4~9位将被掩码处理。
首先,我们需要编写一个 Mapper 函数,它将生成一条记录并将其写入标准输出。Mapper 函数的输入参数是无关紧要的,因此我们可以将其设置为 `_`。Mapper 函数的输出键是 `NullWritable.get()`,值是一条记录的字符串表示形式。
```java
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Random;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class DataGeneratorMapper extends Mapper<Object, Text, NullWritable, Text> {
private static final String[] MODELS = {"iPhone", "Samsung", "Huawei", "Xiaomi", "Oppo", "Vivo"};
private static final String[] OS_LIST = {"iOS", "Android"};
private static final String PHONE_PREFIX = "138";
private static final String PHONE_MASK = "******";
private static final SimpleDateFormat DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private static final Random RANDOM = new Random();
private Text outputValue = new Text();
@Override
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// 生成id
int recordId = context.getTaskAttemptID().getTaskID().getId() * 1000 + RANDOM.nextInt(1000);
// 生成日期
String recordDate = DATE_FORMAT.format(new Date());
// 生成手机号码
String phoneSuffix = String.format("%04d", RANDOM.nextInt(10000));
String phoneNumber = PHONE_PREFIX + PHONE_MASK + phoneSuffix;
// 生成手机型号和操作系统
String model = MODELS[RANDOM.nextInt(MODELS.length)];
String os = OS_LIST[RANDOM.nextInt(OS_LIST.length)];
// 构造输出字符串
String outputString = String.format("%d,%s,%s,%s,%s", recordId, recordDate, phoneNumber, model, os);
outputValue.set(outputString);
// 输出键值对
context.write(NullWritable.get(), outputValue);
}
}
```
然后,我们需要编写一个 Reducer 函数,它将 Mapper 函数输出的所有记录都写入一个文件。Reducer 函数的输入参数是 Mapper 函数输出的键值对,输出类型是 `NullWritable` 和 `Text`,即不输出键,只输出值。
```java
import java.io.IOException;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class DataGeneratorReducer extends Reducer<NullWritable, Text, NullWritable, Text> {
@Override
public void reduce(NullWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// 将所有记录写入文件
for (Text value : values) {
context.write(NullWritable.get(), value);
}
}
}
```
最后,我们需要编写一个驱动程序,它将 Mapper 函数和 Reducer 函数组合起来,并将它们提交到 Hadoop 集群上运行。驱动程序的主要作用是设置 MapReduce 作业的配置参数,例如输入路径、输出路径、Mapper 函数、Reducer 函数、输出键值对类型等。
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import org.apache.hadoop.mapreduce.lib.output.TextOutputFormat;
public class DataGeneratorDriver {
public static void main(String[] args) throws Exception {
if (args.length != 2) {
System.err.println("Usage: DataGeneratorDriver <outputPath> <numRecords>");
System.exit(1);
}
String outputPath = args[0];
int numRecords = Integer.parseInt(args[1]);
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "DataGenerator");
job.setJarByClass(DataGeneratorDriver.class);
job.setMapperClass(DataGeneratorMapper.class);
job.setReducerClass(DataGeneratorReducer.class);
job.setOutputKeyClass(NullWritable.class);
job.setOutputValueClass(Text.class);
job.setOutputFormatClass(TextOutputFormat.class);
// 设置输出路径
FileOutputFormat.setOutputPath(job, new Path(outputPath));
// 设置输出文件大小
FileOutputFormat.setMinOutputFileSize(job, numRecords / 10);
FileOutputFormat.setMaxOutputFileSize(job, numRecords);
// 提交作业并等待完成
job.waitForCompletion(true);
}
}
```
在命令行中运行以下命令来启动数据生成器作业:
```
hadoop jar datagenerator.jar DataGeneratorDriver <outputPath> <numRecords>
```
其中,`datagenerator.jar` 是包含驱动程序和依赖项的 JAR 文件,`<outputPath>` 是输出路径,`<numRecords>` 是要生成的记录数。根据指定的记录数,Hadoop 会自动计算输出文件的大小,并将所有记录写入一个或多个文件中。
相关推荐
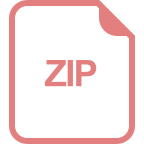
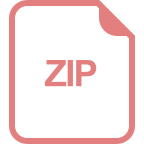
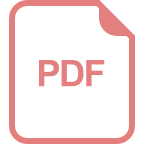














