基于51单片机的温度检测系统设计
时间: 2023-07-26 16:15:19 浏览: 122
以下是一个基于51单片机的温度检测系统的设计方案:
硬件设计:
1. AT89S52单片机
2. DS18B20数字温度传感器
3. 16x2 LCD液晶显示屏
4. 4.7K欧姆电阻
5. 9V电源
6. 杜邦线和面包板
软件设计:
1. Keil C51编译器
2. Proteus仿真软件
基本思路:
1. 将DS18B20数字温度传感器连接到单片机上,并进行初始化设置。
2. 单片机启动后,通过读取DS18B20传感器的数据获取当前环境温度。
3. 将温度数据显示在LCD液晶显示屏上。
4. 通过串口通信将温度数据发送到上位机,实现远程监控。
5. 使用电路连接线和电阻,将整个系统连接到9V电源模块上,以保证系统正常运行。
6. 使用Keil C51编译器编写程序,实现系统逻辑和功能。
7. 使用Proteus仿真软件进行系统仿真和测试。
以下是一个基于51单片机的温度检测系统的程序代码:
```c
#include <reg52.h>
#include <stdio.h>
#include <string.h>
sbit DQ = P3^7; // DS18B20数据线连接到P3.7
sbit RS = P2^0; // LCD液晶显示屏RS引脚连接到P2.0
sbit RW = P2^1; // LCD液晶显示屏RW引脚连接到P2.1
sbit EN = P2^2; // LCD液晶显示屏EN引脚连接到P2.2
void Delay1ms(unsigned int i) // 延时函数
{
unsigned int j;
while(i--)
{
for(j=0;j<120;j++);
}
}
void LCD_Write_Cmd(unsigned char cmd) // 写指令函数
{
RW = 0;
RS = 0;
P0 = cmd;
EN = 1;
Delay1ms(5);
EN = 0;
}
void LCD_Write_Data(unsigned char dat) // 写数据函数
{
RW = 0;
RS = 1;
P0 = dat;
EN = 1;
Delay1ms(5);
EN = 0;
}
void LCD_Init() // LCD初始化函数
{
LCD_Write_Cmd(0x38);
LCD_Write_Cmd(0x0c);
LCD_Write_Cmd(0x06);
LCD_Write_Cmd(0x01);
}
void LCD_Write_String(unsigned char x, unsigned char y, unsigned char *s) // 在LCD上显示字符串函数
{
unsigned char i;
if(x<16)
{
if(y) x |= 0x40;
x |= 0x80;
LCD_Write_Cmd(x);
}
i = 0;
while(s[i])
{
LCD_Write_Data(s[i]);
i++;
}
}
unsigned char DS18B20_Reset() // DS18B20复位函数
{
unsigned char i;
DQ = 1;
Delay1ms(1);
DQ = 0;
Delay1ms(480);
DQ = 1;
Delay1ms(60);
i = DQ;
Delay1ms(420);
return i;
}
void DS18B20_Write_Byte(unsigned char dat) // DS18B20写字节函数
{
unsigned char i;
for(i=0;i<8;i++)
{
DQ = 0;
DQ = dat & 0x01;
Delay1ms(5);
DQ = 1;
dat >>= 1;
}
}
unsigned char DS18B20_Read_Byte() // DS18B20读字节函数
{
unsigned char i, dat = 0;
for(i=0;i<8;i++)
{
DQ = 0;
Delay1ms(5);
DQ = 1;
dat >>= 1;
if(DQ) dat |= 0x80;
Delay1ms(5);
}
return dat;
}
void DS18B20_Start() // DS18B20开始转换函数
{
DS18B20_Reset();
DS18B20_Write_Byte(0xcc);
DS18B20_Write_Byte(0x44);
}
float DS18B20_Read_Temp() // DS18B20读温度函数
{
unsigned char temp_l, temp_h;
float temp;
DS18B20_Reset();
DS18B20_Write_Byte(0xcc);
DS18B20_Write_Byte(0xbe);
temp_l = DS18B20_Read_Byte();
temp_h = DS18B20_Read_Byte();
temp = temp_h;
temp *= 256;
temp += temp_l;
temp /= 16;
return temp;
}
void main()
{
float temp;
unsigned char str[16];
LCD_Init();
while(1)
{
DS18B20_Start();
temp = DS18B20_Read_Temp();
sprintf(str, "Temp:%.2fC", temp);
LCD_Write_String(0, 0, str);
Delay1ms(1000);
}
}
```
该代码中使用了DS18B20数字温度传感器和LCD液晶显示屏。需要注意的是,由于51单片机没有内置的ADC模块,因此我们选择了DS18B20数字温度传感器,需要使用DS18B20相关函数进行温度值的读取。同时,LCD液晶显示屏的初始化也需要在程序中进行。
阅读全文
相关推荐
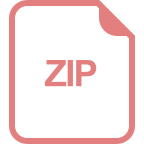
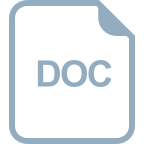
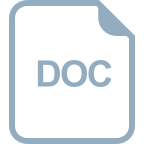
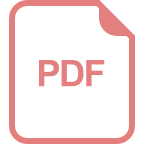
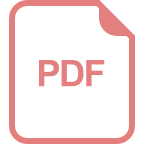
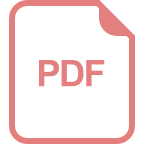
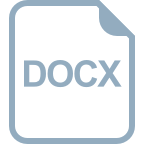
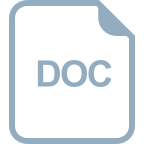
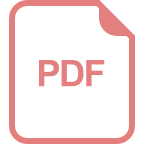
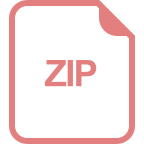
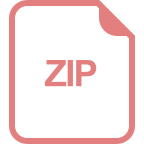
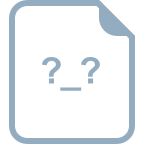
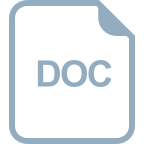
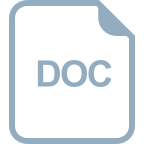