vue3 props 设置type 为 boolean, 然后传入undefined
时间: 2024-05-06 12:16:53 浏览: 211
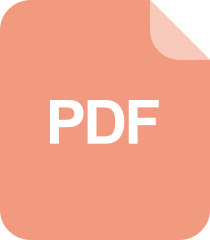
vue中使用props传值的方法

在 Vue3 中,如果你将一个 prop 的 type 设置为 boolean,但是传入 undefined,那么它会被视为一个有效的值,但是它的值将是 undefined。这意味着你的组件可以接受一个未定义的布尔值,并且不会引发类型错误。
例如,假设你有一个组件:
```
<template>
<div>{{ show }}</div>
</template>
<script>
export default {
props: {
show: {
type: Boolean
}
}
}
</script>
```
如果你将 show 属性传入 undefined:
```
<my-component :show="undefined" />
```
那么组件将会渲染为:
```
<div>undefined</div>
```
因此,你需要在组件中检查 show 属性是否是 undefined,并根据需要采取适当的措施。
阅读全文
相关推荐
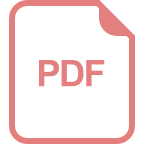
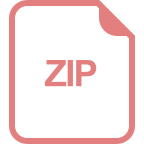
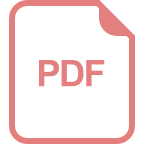
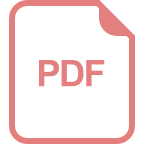
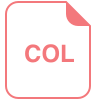
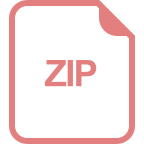
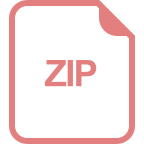
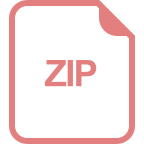
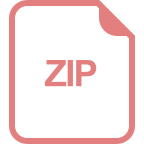