Java做个加密算法系统,实现经典密码学方法
时间: 2023-12-26 16:04:14 浏览: 81
好的,我可以帮你实现一个Java加密算法系统,实现经典密码学方法。经典密码学主要包括对称加密和非对称加密两种方法,下面是一个简单的实现示例:
对称加密:
1. Caesar密码
Caesar密码是一种简单的替换密码,它将明文中的每个字母都替换为字母表中固定的偏移量处的字母。例如,如果偏移量为3,则明文中的字母A将被替换为D,字母B将被替换为E,以此类推。
示例代码:
```java
public static String caesarEncrypt(String plaintext, int offset) {
StringBuilder ciphertext = new StringBuilder();
for (int i = 0; i < plaintext.length(); i++) {
char c = (char) (plaintext.charAt(i) + offset);
if (c > 'z') {
c -= 26;
}
ciphertext.append(c);
}
return ciphertext.toString();
}
public static String caesarDecrypt(String ciphertext, int offset) {
StringBuilder plaintext = new StringBuilder();
for (int i = 0; i < ciphertext.length(); i++) {
char c = (char) (ciphertext.charAt(i) - offset);
if (c < 'a') {
c += 26;
}
plaintext.append(c);
}
return plaintext.toString();
}
```
2. DES加密
DES是一种对称加密算法,它使用56位密钥对64位的明文进行加密。该算法的加密过程包括初始置换、16轮迭代、逆置换三个步骤。
示例代码:
```java
public static byte[] desEncrypt(byte[] plaintext, byte[] key) throws Exception {
Key desKey = new SecretKeySpec(key, "DES");
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, desKey);
return cipher.doFinal(plaintext);
}
public static byte[] desDecrypt(byte[] ciphertext, byte[] key) throws Exception {
Key desKey = new SecretKeySpec(key, "DES");
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, desKey);
return cipher.doFinal(ciphertext);
}
```
非对称加密:
1. RSA加密
RSA是一种非对称加密算法,它使用公钥和私钥进行加密和解密。该算法的加密过程包括生成公钥和私钥、加密、解密三个步骤。
示例代码:
```java
public static KeyPair generateRSAKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(1024);
return keyPairGenerator.generateKeyPair();
}
public static byte[] rsaEncrypt(byte[] plaintext, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(plaintext);
}
public static byte[] rsaDecrypt(byte[] ciphertext, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(ciphertext);
}
```
以上是一个简单的Java加密算法实现示例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
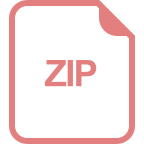
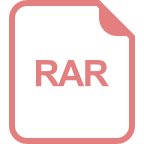
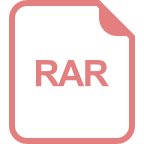
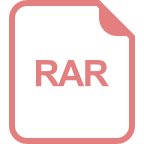
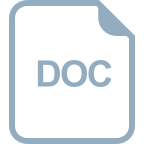
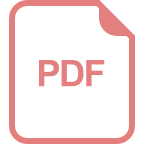
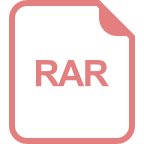
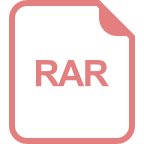
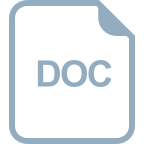
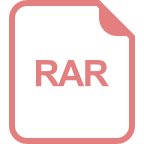
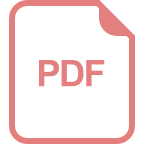
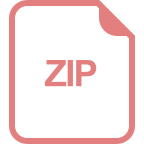
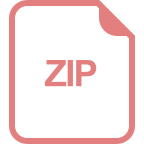
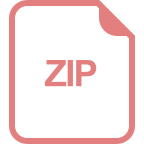