你上面写的用不了,再写一个
时间: 2024-03-21 18:38:20 浏览: 78
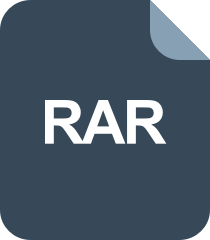
前面的有问题,这个可以
非常抱歉,我的回答不能正常工作。以下是更详细的教程和代码:
首先,确保场景中存在 NavMesh。如果没有,请使用 Unity 的 Navigation 工具创建一个 NavMesh。
接下来,在场景中创建一个空物体并将其命名为 Enemy。将 NavMeshAgent 组件添加到 Enemy 上,并将其设置为自动更新。
现在,我们需要创建一个脚本来控制 Enemy 的行为。创建一个新的 C# 脚本并将其命名为 EnemyController。
在 EnemyController 脚本中创建以下变量:
```csharp
public float moveSpeed = 3f;
public float stoppingDistance = 0.1f;
public float waitTime = 3f;
private NavMeshAgent agent;
private Vector3[] waypoints;
private int currentWaypointIndex = 0;
private bool isWaiting = false;
private float waitTimer = 0f;
```
- moveSpeed:敌人的移动速度。
- stoppingDistance:敌人到达目标点后停止的距离。
- waitTime:敌人在到达目标点后等待的时间。
- agent:NavMeshAgent 组件的引用。
- waypoints:敌人需要巡逻的目标点列表。
- currentWaypointIndex:当前目标点的索引。
- isWaiting:敌人是否在等待。
- waitTimer:等待计时器。
在 Start 函数中,我们需要初始化 NavMeshAgent 组件并生成巡逻目标点。我们可以使用 Random.insideUnitSphere 函数生成一个随机位置,并使用 NavMesh.SamplePosition 函数将其投射到 NavMesh 上。我们可以重复这个过程来生成多个随机位置,并将它们存储在 waypoints 数组中。
```csharp
void Start()
{
agent = GetComponent<NavMeshAgent>();
agent.speed = moveSpeed;
// 生成随机目标点列表
waypoints = new Vector3[5];
for (int i = 0; i < waypoints.Length; i++)
{
Vector3 randomPosition = transform.position + Random.insideUnitSphere * 10f;
NavMesh.SamplePosition(randomPosition, out NavMeshHit hit, 10f, NavMesh.AllAreas);
waypoints[i] = hit.position;
}
// 设置第一个目标点
agent.SetDestination(waypoints[0]);
}
```
在 Update 函数中,我们需要检查敌人是否到达了当前目标点。如果到达了,我们需要等待一段时间后选择下一个目标点。我们可以使用 Vector3.Distance 函数来计算敌人与目标点之间的距离。如果距离小于 stoppingDistance,我们将设置 isWaiting 为 true 并开始计时。如果等待时间超过 waitTime,我们将选择下一个目标点并重新开始移动。
```csharp
void Update()
{
if (isWaiting)
{
waitTimer += Time.deltaTime;
if (waitTimer >= waitTime)
{
isWaiting = false;
waitTimer = 0f;
// 选择下一个目标点
currentWaypointIndex++;
if (currentWaypointIndex >= waypoints.Length)
{
currentWaypointIndex = 0;
}
// 移动到下一个目标点
agent.SetDestination(waypoints[currentWaypointIndex]);
}
}
else
{
if (agent.remainingDistance <= agent.stoppingDistance)
{
isWaiting = true;
}
}
}
```
最后,我们需要将 EnemyController 脚本添加到 Enemy 上并运行游戏。Enemy 将开始随机巡逻场景中的目标点。
完整的 EnemyController 脚本如下所示:
```csharp
using UnityEngine;
using UnityEngine.AI;
public class EnemyController : MonoBehaviour
{
public float moveSpeed = 3f;
public float stoppingDistance = 0.1f;
public float waitTime = 3f;
private NavMeshAgent agent;
private Vector3[] waypoints;
private int currentWaypointIndex = 0;
private bool isWaiting = false;
private float waitTimer = 0f;
void Start()
{
agent = GetComponent<NavMeshAgent>();
agent.speed = moveSpeed;
// 生成随机目标点列表
waypoints = new Vector3[5];
for (int i = 0; i < waypoints.Length; i++)
{
Vector3 randomPosition = transform.position + Random.insideUnitSphere * 10f;
NavMesh.SamplePosition(randomPosition, out NavMeshHit hit, 10f, NavMesh.AllAreas);
waypoints[i] = hit.position;
}
// 设置第一个目标点
agent.SetDestination(waypoints[0]);
}
void Update()
{
if (isWaiting)
{
waitTimer += Time.deltaTime;
if (waitTimer >= waitTime)
{
isWaiting = false;
waitTimer = 0f;
// 选择下一个目标点
currentWaypointIndex++;
if (currentWaypointIndex >= waypoints.Length)
{
currentWaypointIndex = 0;
}
// 移动到下一个目标点
agent.SetDestination(waypoints[currentWaypointIndex]);
}
}
else
{
if (agent.remainingDistance <= agent.stoppingDistance)
{
isWaiting = true;
}
}
}
}
```
阅读全文
相关推荐
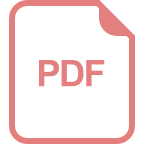
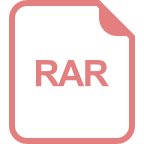
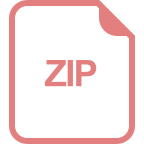
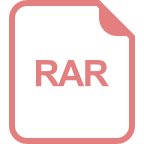
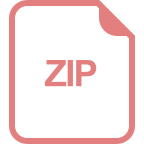
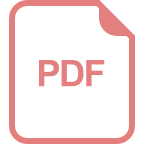
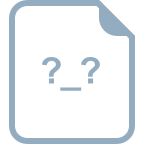
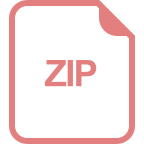
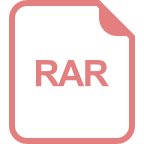
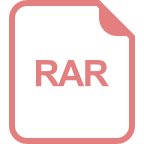
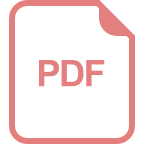
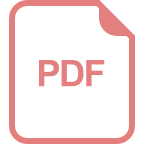
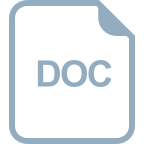
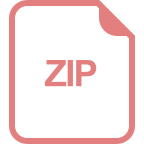
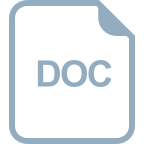
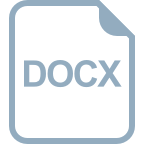