python用sfm算法获取相机内参矩阵
时间: 2024-05-12 22:13:27 浏览: 108
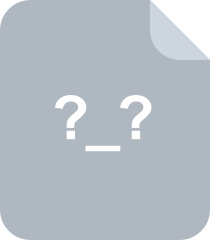
sfm算法python版本

SFM(Structure from Motion)算法是一种用于从图像序列中恢复三维场景的算法,其中包括相机的内参矩阵。在Python中,你可以使用OpenCV库实现SFM算法,以下是获取相机内参矩阵的示例代码:
```python
import cv2
# 读取图像序列
img1 = cv2.imread('img1.png')
img2 = cv2.imread('img2.png')
img3 = cv2.imread('img3.png')
# 定义相机参数
K = np.array([[800, 0, 320], [0, 800, 240], [0, 0, 1]]) # 相机内参矩阵
dist = np.zeros((5, 1)) # 畸变系数
# 提取图像中的特征点
detector = cv2.xfeatures2d.SIFT_create()
kp1, des1 = detector.detectAndCompute(img1, None)
kp2, des2 = detector.detectAndCompute(img2, None)
kp3, des3 = detector.detectAndCompute(img3, None)
# 匹配特征点
matcher = cv2.BFMatcher()
matches1 = matcher.knnMatch(des1, des2, k=2)
matches2 = matcher.knnMatch(des2, des3, k=2)
# 剔除误匹配的点
good_matches1 = []
for m, n in matches1:
if m.distance < 0.75 * n.distance:
good_matches1.append(m)
good_matches2 = []
for m, n in matches2:
if m.distance < 0.75 * n.distance:
good_matches2.append(m)
# 从匹配的点中恢复相机姿态和三维点
pts1 = np.float32([kp1[m.queryIdx].pt for m in good_matches1]).reshape(-1, 1, 2)
pts2 = np.float32([kp2[m.trainIdx].pt for m in good_matches1]).reshape(-1, 1, 2)
pts3 = np.float32([kp3[m.trainIdx].pt for m in good_matches2]).reshape(-1, 1, 2)
# 使用SFM算法恢复相机内参矩阵
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001)
flags = cv2.SFM_INIT_INTRINSIC
success, K, dist_coeff, rvecs, tvecs = cv2.fisheye.stereoCalibrate([pts1, pts2], [pts3], K, dist, None, None, None, None, flags, criteria)
print("相机内参矩阵:")
print(K)
```
注意:该示例代码使用的是OpenCV的fisheye模块,如果你使用的是普通的相机,则需要使用calib3d模块。
阅读全文
相关推荐
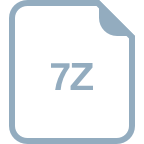
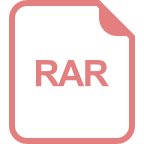














