一维卷积神经网络光谱预测代码
时间: 2023-09-25 15:15:50 浏览: 48
我可以提供一段简单的一维卷积神经网络光谱预测代码示例:
```
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# Define the one-dimensional convolutional neural network model
class SpectrumCNN(nn.Module):
def __init__(self):
super(SpectrumCNN, self).__init__()
self.conv1d_1 = nn.Conv1d(1, 32, kernel_size=3, stride=1, padding=1)
self.conv1d_2 = nn.Conv1d(32, 64, kernel_size=3, stride=1, padding=1)
self.pool = nn.MaxPool1d(kernel_size=2, stride=2)
self.fc1 = nn.Linear(64 * 50, 128)
self.fc2 = nn.Linear(128, 1)
def forward(self, x):
x = x.unsqueeze(1)
x = self.pool(nn.functional.relu(self.conv1d_1(x)))
x = self.pool(nn.functional.relu(self.conv1d_2(x)))
x = x.view(-1, 64 * 50)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# Generate some synthetic data for training the model
X = np.random.rand(1000, 50)
y = np.sum(X, axis=1, keepdims=True)
# Convert the data to PyTorch tensors
X = torch.tensor(X, dtype=torch.float32)
y = torch.tensor(y, dtype=torch.float32)
# Create a SpectrumCNN model and an optimizer
model = SpectrumCNN()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Train the model for 100 epochs
for epoch in range(100):
optimizer.zero_grad()
output = model(X)
loss = nn.functional.mse_loss(output, y)
loss.backward()
optimizer.step()
print("Epoch %d, Loss=%.3f" % (epoch + 1, loss.item()))
# Test the model on some new data
X_test = np.random.rand(10, 50)
y_test = np.sum(X_test, axis=1, keepdims=True)
X_test = torch.tensor(X_test, dtype=torch.float32)
y_test = torch.tensor(y_test, dtype=torch.float32)
pred = model(X_test)
print("Predictions:", pred.detach().numpy().flatten())
print("True Values:", y_test.detach().numpy().flatten())
```
这段代码创建了一个名为 SpectrumCNN 的一维卷积神经网络模型,用于预测具有 50 个特征的光谱数据。模型包括两个卷积层和两个全连接层,用于将输入数据映射到输出数据。模型在 MSE 损失函数下进行训练,并使用 Adam 优化器来更新模型参数。最后,模型在一些新数据上进行测试,并将预测值与真实值打印出来。
相关推荐
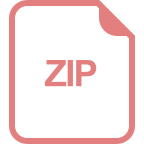
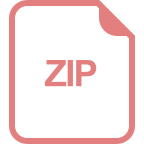
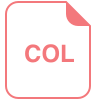













