c++建立一个线性表
时间: 2023-07-01 21:20:25 浏览: 101
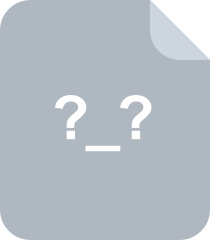
c++实现线性表

在C++中可以使用数组或链表来实现线性表。下面是使用数组实现线性表的示例代码:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 线性表的最大长度
class SeqList {
private:
int data[MAXSIZE]; // 存储元素的数组
int length; // 线性表的长度
public:
SeqList() {
length = 0; // 初始化线性表的长度为0
}
int getLength() {
return length;
}
int getElem(int i) {
if (i < 1 || i > length) {
cout << "Error: index out of range!" << endl;
return -1; // 返回一个错误值
}
return data[i-1];
}
void insert(int i, int elem) {
if (i < 1 || i > length + 1 || length >= MAXSIZE) {
cout << "Error: insertion failed!" << endl;
return;
}
for (int j = length; j >= i; j--) {
data[j] = data[j-1];
}
data[i-1] = elem;
length++;
}
void remove(int i) {
if (i < 1 || i > length) {
cout << "Error: deletion failed!" << endl;
return;
}
for (int j = i; j < length; j++) {
data[j-1] = data[j];
}
length--;
}
void display() {
cout << "The elements in the list are: ";
for (int i = 0; i < length; i++) {
cout << data[i] << " ";
}
cout << endl;
}
};
int main() {
SeqList list;
list.insert(1, 10);
list.insert(2, 20);
list.insert(3, 30);
list.display(); // 输出:The elements in the list are: 10 20 30
list.remove(2);
list.display(); // 输出:The elements in the list are: 10 30
cout << "The length of the list is: " << list.getLength() << endl; // 输出:The length of the list is: 2
cout << "The element at position 2 is: " << list.getElem(2) << endl; // 输出:The element at position 2 is: 30
return 0;
}
```
以上代码使用了类的封装,包含了线性表的常见操作:获取长度、获取元素、插入元素、删除元素、显示所有元素。你可以根据需求进行修改和扩展。
阅读全文
相关推荐



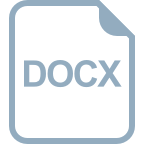











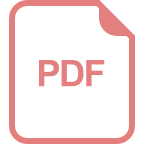