逐行解释该C语言代码:#include<iostream> using namespace std; int merge(int *a,int low,int mid,int high,int count) { int b[high+1]; int h,i,j,k; count=0; h=low; j=mid+1; i=low; while(h<=mid && j<=high) { if(a[h]<=2*a[j]) { b[i]=a[h]; h=h+1; } else { count=count+mid-h+1; b[i]=a[j]; j=j+1; } i=i+1; } if(h>mid) { for(k=j;k<=high;k++) { b[i]=a[k]; i=i+1; } } else { for(k=h;k<=mid;k++) { b[i]=a[k]; i=i+1; } } for(k=low;k<=high;k++) { a[k]=b[k]; } return count; } int find(int *a,int low,int high,int count) { int mid,count1=0,count2=0,count3=0; if(high<=low) return 0; else { mid=(low+high)/2; count1=find(a,low,mid,count1); count2=find(a,mid+1,high,count2); count3=merge(a,low,mid,high,count3); return count1+count2+count3; } } int main() { char c[10000]; int a[10000]; int i=0; int j=0; while(cin>>c[i]) { if(c[i]>='0'&&c[i]<='9') { a[j]=c[i]-'0'; j++; } if(c[i]==']') break; i++; } int count=find(a,0,j-1,0); printf("%d\n",count); return 0; }
时间: 2024-02-01 10:03:37 浏览: 63
这段代码实现了一个归并排序,其中 `merge` 函数将两个有序的数组合并为一个有序数组,`find` 函数实现了分治法,将数组不断分为两个子数组,然后再使用 `merge` 函数将它们合并,最终统计出逆序对的个数。下面逐行解释这段代码:
```
#include<iostream>
using namespace std;
int merge(int *a,int low,int mid,int high,int count)
{
int b[high+1];
int h,i,j,k;
count=0;
h=low;
j=mid+1;
i=low;
while(h<=mid && j<=high)
{
if(a[h]<=2*a[j])
{
b[i]=a[h];
h=h+1;
}
else
{
count=count+mid-h+1;
b[i]=a[j];
j=j+1;
}
i=i+1;
}
if(h>mid)
{
for(k=j;k<=high;k++)
{
b[i]=a[k];
i=i+1;
}
}
else
{
for(k=h;k<=mid;k++)
{
b[i]=a[k];
i=i+1;
}
}
for(k=low;k<=high;k++)
{
a[k]=b[k];
}
return count;
}
```
`merge` 函数实现了将两个有序数组 `a[low:mid]` 和 `a[mid+1:high]` 合并为一个有序数组,并计算逆序对的个数。其中 `count` 是逆序对的计数器,`b` 数组是用于储存排序后的数组。在函数体中,`h`、`i`、`j` 和 `k` 是循环计数器,`low`、`mid` 和 `high` 是数组下标的界限。
```
int find(int *a,int low,int high,int count)
{
int mid,count1=0,count2=0,count3=0;
if(high<=low)
return 0;
else
{
mid=(low+high)/2;
count1=find(a,low,mid,count1);
count2=find(a,mid+1,high,count2);
count3=merge(a,low,mid,high,count3);
return count1+count2+count3;
}
}
```
`find` 函数实现了分治法,将数组不断分为两个子数组,然后再使用 `merge` 函数将它们合并,最终统计出逆序对的个数。其中 `count1`、`count2` 和 `count3` 都是逆序对的计数器,`mid` 是数组的中间位置,`low` 和 `high` 是数组下标的界限。
```
int main()
{
char c[10000];
int a[10000];
int i=0;
int j=0;
while(cin>>c[i])
{
if(c[i]>='0'&&c[i]<='9')
{
a[j]=c[i]-'0';
j++;
}
if(c[i]==']')
break;
i++;
}
int count=find(a,0,j-1,0);
printf("%d\n",count);
return 0;
}
```
`main` 函数读入一个包含整数的数组,并调用 `find` 函数统计逆序对的个数,最终输出结果。其中 `c` 数组用于储存输入字符,`a` 数组用于储存整数,`i` 和 `j` 是循环计数器。这段代码使用了 C++ 的 `iostream` 库和命名空间 `std`,还使用了 C 的标准输入输出库 `stdio.h` 中的 `printf` 函数。
阅读全文
相关推荐
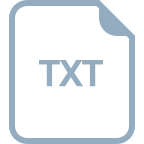
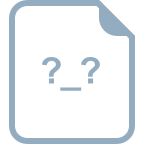
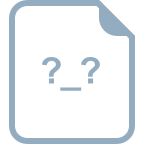
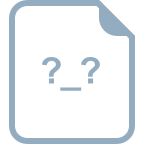
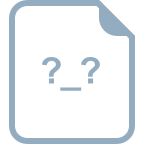
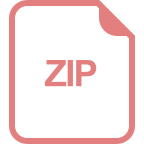
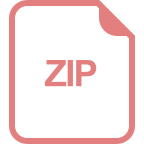
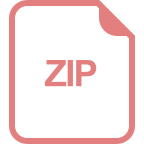
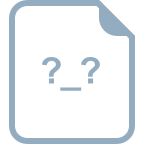
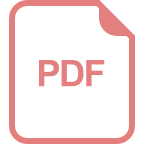
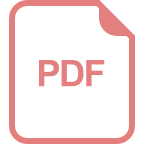
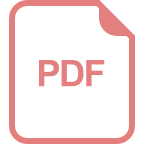
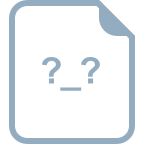
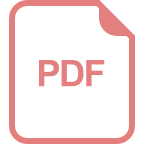
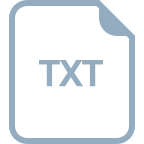
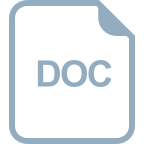
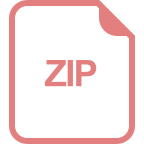
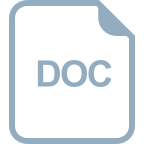
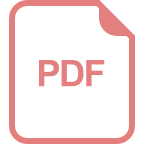