VUE v-for循环数组arr,div中显示对象中的某一值item.name,div横向排列,超出设定的长度时,div则自动换行,点击某一item.name时如何确定点击的元素是第几行的,点击item.name时需要向下展开一个div,如何在点击第一个item.name的时候展开div,再次点击此item.name时收起,点击其他的item.name则切换成对应的div内容,只有点击第一个item.name才能收起
时间: 2024-02-28 15:53:34 浏览: 97
对于第一个问题,你可以这样实现:
```html
<div id="app">
<div v-for="(item, index) in arr" :key="index" class="item" @click="handleClick(index)">
{{ item.name }}
</div>
</div>
```
```css
.item {
display: inline-block;
margin-right: 20px;
margin-bottom: 20px;
}
```
```js
new Vue({
el: '#app',
data: {
arr: [
{ name: 'A' },
{ name: 'B' },
{ name: 'C' },
{ name: 'D' },
{ name: 'E' },
{ name: 'F' },
{ name: 'G' },
{ name: 'H' },
{ name: 'I' },
{ name: 'J' },
{ name: 'K' },
{ name: 'L' }
],
selectedIndex: -1
},
methods: {
handleClick(index) {
if (this.selectedIndex === index) {
this.selectedIndex = -1;
} else {
this.selectedIndex = index;
}
}
}
})
```
对于第二个问题,你可以在每个 item 中添加一个子元素,并根据 `selectedIndex` 控制其显示或隐藏,示例代码如下:
```html
<div id="app">
<div v-for="(item, index) in arr" :key="index" class="item" @click="handleClick(index)">
{{ item.name }}
<div class="sub" v-if="selectedIndex === index">
sub content
</div>
</div>
</div>
```
```css
.item {
display: inline-block;
margin-right: 20px;
margin-bottom: 20px;
}
.sub {
display: none;
width: 100px;
height: 100px;
background-color: #ccc;
position: absolute;
top: 100%;
left: 0;
}
.sub.show {
display: block;
}
```
```js
new Vue({
el: '#app',
data: {
arr: [
{ name: 'A' },
{ name: 'B' },
{ name: 'C' },
{ name: 'D' },
{ name: 'E' },
{ name: 'F' },
{ name: 'G' },
{ name: 'H' },
{ name: 'I' },
{ name: 'J' },
{ name: 'K' },
{ name: 'L' }
],
selectedIndex: -1
},
methods: {
handleClick(index) {
const subElements = document.querySelectorAll('.sub');
subElements.forEach((item, i) => {
if (i === index) {
item.classList.toggle('show');
} else {
item.classList.remove('show');
}
});
if (this.selectedIndex === index) {
this.selectedIndex = -1;
} else {
this.selectedIndex = index;
}
}
}
})
```
这样,点击某个 item 的时候,对应的子元素就会显示或隐藏,并且只有点击第一个 item 才能收起。
阅读全文
相关推荐
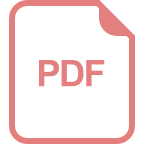
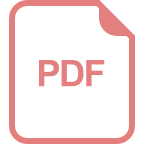
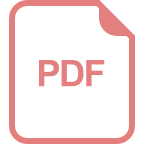
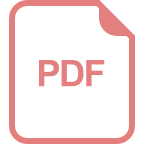
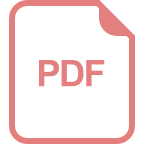
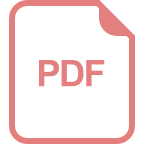
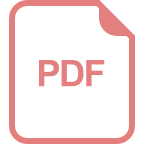
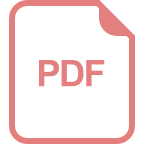
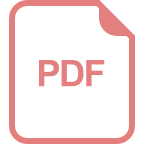
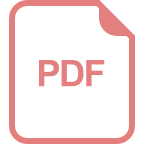
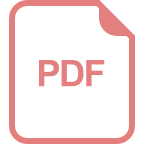
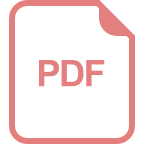
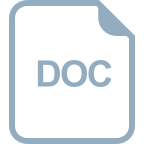
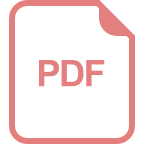