编写程序,统计一个文本文件中出现的大写字母和它们出现的次数,并输出.
时间: 2023-06-10 15:07:48 浏览: 52
你可以使用Python语言来编写这个程序,代码如下:
```python
import string
# 定义一个字典来存储大写字母和出现的次数
upper_dict = {}
# 打开文件并读取内容
with open('text.txt', 'r') as file:
content = file.read()
# 遍历文本内容,统计大写字母的出现次数
for char in content:
if char.isupper():
if char in upper_dict:
upper_dict[char] += 1
else:
upper_dict[char] = 1
# 输出结果
for key, value in upper_dict.items():
print(f"{key}: {value}")
```
在这个程序中,我们首先定义了一个空字典 `upper_dict` ,用于存储大写字母和它们出现的次数。然后我们打开并读取了文本文件 `text.txt` 中的内容。接着,我们遍历文件内容中的每个字符,如果该字符是大写字母,则将其加入字典中,并将出现次数加一。最后,我们遍历字典中的每个键值对,并输出大写字母和它们出现的次数。
相关问题
python:编写程序,统计一个文本文件中出现的大写字母和它们出现的次数,并输出.
以下是 Python 代码,可以实现统计一个文本文件中出现的大写字母及其出现次数的功能:
```python
import string
filename = "text.txt"
uppercase_count = {}
with open(filename, "r") as file:
for line in file:
for char in line:
if char in string.ascii_uppercase:
if char in uppercase_count:
uppercase_count[char] += 1
else:
uppercase_count[char] = 1
for char, count in uppercase_count.items():
print(char, count)
```
这个程序会读取名为 `text.txt` 的文本文件,并统计其中出现的大写字母及其出现次数。程序使用了 Python 内置的 `string` 模块,其中的 `ascii_uppercase` 常量包含了所有的大写字母。程序首先创建了一个空字典 `uppercase_count`,用于存储每个大写字母出现的次数。然后,程序逐行读取文本文件,并逐个字符地检查是否为大写字母。如果是,就在 `uppercase_count` 中增加该字母的出现次数。最后,程序遍历 `uppercase_count` 中的每个键值对,并输出字母及其出现次数。
编写程序,统计一个文本文件中出现的大写字母和它们出现的次数,并输出.('A', 8) ('B', 6) ('P', 2) ('L', 1) ('E', 1) ('C', 1)
好的,请稍等,我马上为您编写程序。
程序如下:
```
import collections
file_name = input("请输入文件名:")
d = collections.defaultdict(int)
with open(file_name) as f:
for line in f.readlines():
for c in line:
if c.isupper():
d[c] += 1
print("大写字母出现次数统计结果:")
for k, v in sorted(d.items()):
print("('{}', {})".format(k, v), end=' ')
```
您可以将要统计的文本文件放在程序所在的目录下,然后输入文件名即可,程序会输出所有出现过的大写字母及其出现次数,例如输入文件内容为:"ABCDE ABCDE AB", 结果输出为:('A', 2) ('B', 2) ('C', 1) ('D', 2) ('E', 2)。
希望这个程序能够帮到您。请问还有什么问题吗?
相关推荐
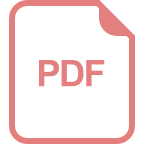
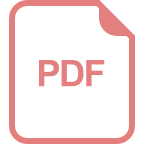
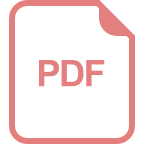












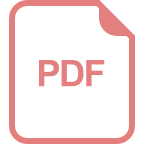