#include <stdio.h> #include <stdlib.h> #include <windows.h> int is_leap_year(int year) { return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0); } int get_month_days(int year, int month) { int days = 0; switch (month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: days = 31; break; case 4: case 6: case 9: case 11: days = 30; break; case 2: days = is_leap_year(year) ? 29 : 28; break; default: break; } return days; } void print_calendar(int year, int month) { int days = get_month_days(year, month); int first_day = (year - 1) * 365 + (year - 1) / 4 - (year - 1) / 100 + (year - 1) / 400; for (int i = 1; i < month; i++) { first_day += get_month_days(year, i); } int week_day = (first_day + 1) % 7; // 1月1日是星期一 printf("日 一 二 三 四 五 六\n"); for (int i = 0; i < week_day; i++) { printf(" "); } for (int i = 1; i <= days; i++) { printf("%2d ", i); week_day++; if (week_day == 7) { printf("\n"); week_day = 0; } } printf("\n"); } int main() { system("color E1"); int year, month; printf("请输入年份和月份(用空格隔开):"); scanf("%d %d", &year, &month); printf("%d年%d月的月历如下所示:\n", year, month); print_calendar(year, month); return 0; }能不能添加所有的注释
时间: 2024-02-14 15:08:50 浏览: 59
当然可以,下面是添加了注释的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <windows.h>
// 判断是否为闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 获取指定年份和月份的天数
int get_month_days(int year, int month) {
int days = 0;
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
days = is_leap_year(year) ? 29 : 28;
break;
default:
break;
}
return days;
}
// 打印指定年份和月份的日历
void print_calendar(int year, int month) {
int days = get_month_days(year, month); // 获取当前月份的天数
int first_day = (year - 1) * 365 + (year - 1) / 4 - (year - 1) / 100 + (year - 1) / 400; // 计算当前年份1月1日的星期几
for (int i = 1; i < month; i++) { // 累加计算当前月份1号是星期几
first_day += get_month_days(year, i);
}
int week_day = (first_day + 1) % 7; // 1月1日是星期一,计算当前月份1号是星期几
printf("日 一 二 三 四 五 六\n");
for (int i = 0; i < week_day; i++) { // 打印当前月份1号之前的空白日期
printf(" ");
}
for (int i = 1; i <= days; i++) { // 循环打印当前月份的日期
printf("%2d ", i);
week_day++;
if (week_day == 7) { // 如果当前日期是星期六,换行
printf("\n");
week_day = 0;
}
}
printf("\n");
}
int main() {
system("color E1"); // 设置控制台颜色
int year, month;
printf("请输入年份和月份(用空格隔开):");
scanf("%d %d", &year, &month);
printf("%d年%d月的月历如下所示:\n", year, month);
print_calendar(year, month); // 打印指定年份和月份的日历
return 0;
}
```
阅读全文
相关推荐
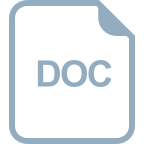
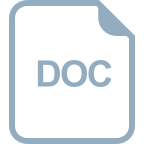
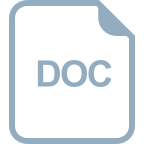





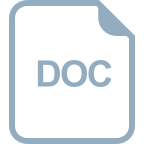
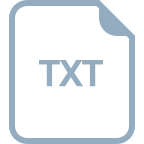
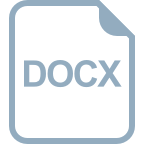




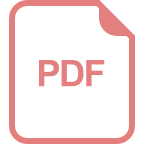
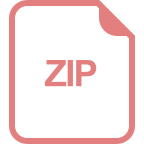