Labwindows使用多线程编程实现串口数据的读写与数据的实时显示
时间: 2023-12-17 15:05:02 浏览: 194
LabWindows/CVI是一个基于Windows的集成开发环境,可以使用其提供的多线程库来实现串口数据的读写和数据的实时显示。
以下是实现的步骤:
1. 打开串口:使用Windows API函数CreateFile打开串口,设置串口参数,并返回串口的句柄。
2. 创建读写线程:使用CVI提供的多线程库函数BeginThreadEx创建读写线程,读线程和写线程分别负责串口数据的读取和写入。
3. 数据处理:读线程读取串口数据后,将数据写入缓冲区,写线程从缓冲区中读取数据,并进行实时显示。
4. 关闭串口:程序结束时,使用Windows API函数CloseHandle关闭串口句柄。
以下是一个简单的LabWindows/CVI程序示例,可以实现串口数据的读写和实时显示:
```c
#include <ansi_c.h>
#include <cvirte.h>
#include <userint.h>
#include <utility.h>
#include <multithread/multithread.h>
#define READ_BUF_SIZE 1024
#define WRITE_BUF_SIZE 1024
int g_readThreadID;
int g_writeThreadID;
int g_stopThread;
HANDLE g_hCom = INVALID_HANDLE_VALUE;
char g_readBuf[READ_BUF_SIZE];
char g_writeBuf[WRITE_BUF_SIZE];
int CVICALLBACK ReadThreadFunc(void *functionData)
{
DWORD dwBytesRead;
while(!g_stopThread)
{
if(ReadFile(g_hCom, g_readBuf, READ_BUF_SIZE, &dwBytesRead, NULL))
{
// 将读取到的数据写入缓冲区
// 可以使用CVI提供的多线程同步机制实现
}
}
return 0;
}
int CVICALLBACK WriteThreadFunc(void *functionData)
{
DWORD dwBytesWritten;
while(!g_stopThread)
{
// 从缓冲区中读取数据,并写入串口
// 可以使用CVI提供的多线程同步机制实现
}
return 0;
}
int main (int argc, char *argv[])
{
// 打开串口
g_hCom = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if(g_hCom == INVALID_HANDLE_VALUE)
{
printf("Open COM failed!\n");
return -1;
}
// 设置串口参数
DCB dcb;
GetCommState(g_hCom, &dcb);
dcb.BaudRate = 9600;
dcb.ByteSize = 8;
dcb.Parity = NOPARITY;
dcb.StopBits = ONESTOPBIT;
SetCommState(g_hCom, &dcb);
// 创建读写线程
g_stopThread = 0;
g_readThreadID = BeginThreadEx(NULL, 0, &ReadThreadFunc, NULL, 0, NULL);
g_writeThreadID = BeginThreadEx(NULL, 0, &WriteThreadFunc, NULL, 0, NULL);
// 实时显示数据
while(!g_stopThread)
{
// 可以使用CVI提供的图形控件实现数据的实时显示
}
// 关闭串口
CloseHandle(g_hCom);
// 终止线程
g_stopThread = 1;
WaitForThreadExit(g_readThreadID, NULL, 0);
WaitForThreadExit(g_writeThreadID, NULL, 0);
return 0;
}
```
注意,在实际应用中,需要使用CVI提供的多线程同步机制来实现读线程和写线程之间的数据交换和共享,以避免多线程同步问题。同时,还需要使用CVI提供的图形控件来实现数据的实时显示。
阅读全文
相关推荐

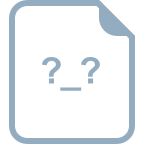












