TypeError: 'numpy.uint8' object is not iterable
时间: 2023-10-25 08:23:31 浏览: 289
这个错误通常出现在尝试对一个 Numpy 数组中的单个元素进行迭代的时候。例如,下面的代码会产生这个错误:
```
import numpy as np
arr = np.array([1, 2, 3])
for i in arr[0]:
print(i)
```
在这个例子中,`arr[0]` 是一个单个的整数,而不是一个数组。因此,当我们尝试对它进行迭代时,Python 报告了一个类型错误。
要避免这个错误,我们需要在迭代之前检查 Numpy 数组的形状。例如,我们可以使用 `numpy.shape` 函数来获取数组的形状,然后检查它是否为单个元素。下面的代码演示了如何这样做:
```
import numpy as np
arr = np.array([1, 2, 3])
if arr.shape == (1,):
print(arr[0])
else:
for i in arr:
print(i)
```
在这个例子中,我们首先检查 `arr.shape` 是否为 `(1,)`。如果是,我们直接打印第一个元素。否则,我们使用一个循环来迭代数组中的每个元素。
相关问题
typeerror: 'numpy.uint8' object is not iterable
这个错误是因为在一个函数或者语句中,把一个 numpy.uint8 类型的对象作为可迭代对象来使用,而 uint8 对象本身是不支持迭代的。需要检查代码,找到使用对象的语句,并确保传入的对象是可以被迭代的数据类型。
TypeError: 'numpy.ndarray' object is not callable
This error occurs when you try to call a numpy array as if it were a function. For example:
```
import numpy as np
my_array = np.array([1, 2, 3])
result = my_array(2)
```
In this example, we are trying to call the numpy array `my_array` with the argument `2`. However, numpy arrays are not callable, and attempting to call them will result in a TypeError.
To fix this error, we need to use the correct syntax for accessing elements of a numpy array:
```
import numpy as np
my_array = np.array([1, 2, 3])
result = my_array[2]
```
Here, we use square brackets to access the third element (index 2) of the numpy array.
阅读全文
相关推荐
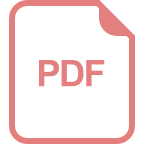

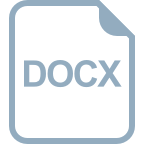
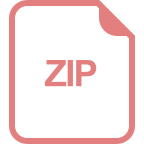
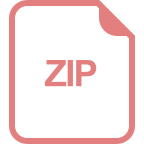
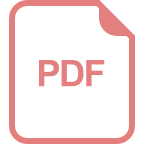