python字符串删除空格
时间: 2023-09-05 12:05:40 浏览: 112
### 回答1:
要删除 Python 字符串中的空格,你可以使用字符串方法 `strip()`。
例如:
```
string = " Hello World! "
string = string.strip()
print(string)
```
输出:
```
Hello World!
```
`strip()` 方法会删除字符串两端的空格,如果你想删除字符串中间的空格,可以使用字符串方法 `replace()`:
```
string = "Hello World!"
string = string.replace(" ", "")
print(string)
```
输出:
```
HelloWorld!
```
如果你想删除字符串所有的空格,可以使用 Python 中的 `join()` 函数:
```
string = " Hello World! "
string = "".join(string.split())
print(string)
```
输出:
```
HelloWorld!
```
### 回答2:
Python中删除字符串中的空格有多种方法。下面是几种常见的方法:
1. 使用 `replace()` 方法来删除空格。可以使用 `replace()` 方法将空格替换为空字符。示例代码如下:
```python
str = "Hello World"
new_str = str.replace(" ", "")
print(new_str) # 输出:HelloWorld
```
2. 使用 `join()` 方法来删除空格。可以使用 `join()` 方法将字符串分割成列表,然后再使用空字符将列表中的元素连接起来。示例代码如下:
```python
str = "Hello World"
new_str = ''.join(str.split())
print(new_str) # 输出:HelloWorld
```
3. 使用正则表达式来删除空格。使用 `re` 模块中的 `sub()` 函数,通过正则表达式匹配空格,将其替换为空字符。示例代码如下:
```python
import re
str = "Hello World"
new_str = re.sub(r'\s', '', str)
print(new_str) # 输出:HelloWorld
```
以上是几种常见的方法,可以根据需求选择合适的方法来删除字符串中的空格。
### 回答3:
在Python中,可以使用strip()方法来删除字符串中的空格。strip()方法是一个字符串方法,可以用于删除字符串开头和结尾的空格。
如果要删除字符串中间的空格,可以将字符串分割成列表,使用''.join()方法将列表中的元素合并成一个字符串。
下面是一个示例代码:
```python
# 删除字符串开头和结尾的空格
string = " Hello, World! "
new_string = string.strip()
print(new_string) # 输出:Hello, World!
# 删除字符串中间的空格
string = "Hello, World!"
string_list = string.split() # 将字符串分割成列表
new_string = ''.join(string_list) # 将列表中的元素合并成一个字符串
print(new_string) # 输出:Hello,World!
```
另外,如果只想删除字符串开头或结尾的空格,可以使用lstrip()方法删除开头的空格,使用rstrip()方法删除结尾的空格。
希望对你有帮助!
相关推荐
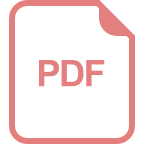
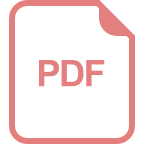
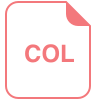
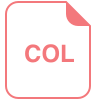
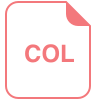
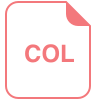
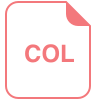









