表单submit后的返回值怎么得到
时间: 2024-03-01 12:51:21 浏览: 22
表单 `submit` 后的返回值可以通过以下两种方式获取:
1. 同步提交方式:在表单 `submit` 事件中,使用 `return` 关键字返回服务器返回的数据。示例代码如下:
```html
<form id="myForm" action="/submit" method="post">
<input type="text" name="username">
<input type="password" name="password">
<button type="submit">提交</button>
</form>
<script>
document.getElementById('myForm').addEventListener('submit', function(event) {
// 阻止表单默认提交行为
event.preventDefault();
// 同步提交表单
var xhr = new XMLHttpRequest();
xhr.open('POST', '/submit', false);
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.send('username=' + this.username.value + '&password=' + this.password.value);
// 返回服务器返回的数据
if (xhr.status === 200) {
console.log(xhr.responseText);
return xhr.responseText;
}
});
</script>
```
需要注意的是,同步提交方式会阻塞页面,不建议使用。
2. 异步提交方式:在表单 `submit` 事件中,使用 AJAX 发送异步请求,通过回调函数获取服务器返回的数据。示例代码如下:
```html
<form id="myForm" action="/submit" method="post">
<input type="text" name="username">
<input type="password" name="password">
<button type="submit">提交</button>
</form>
<script>
document.getElementById('myForm').addEventListener('submit', function(event) {
// 阻止表单默认提交行为
event.preventDefault();
// 异步提交表单
var xhr = new XMLHttpRequest();
xhr.open('POST', '/submit', true);
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send('username=' + this.username.value + '&password=' + this.password.value);
});
</script>
```
异步提交方式需要注意的是,在回调函数中获取的服务器返回的数据是异步获取的,需要在回调函数中进行相应的逻辑处理。
相关推荐
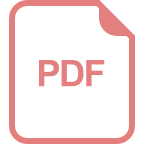
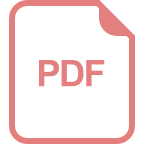
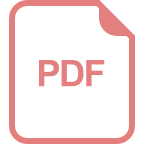














