基于MATLAB的HOG+SVM目标检测案例举例,包含完整的代码及代码讲解
时间: 2024-03-05 10:48:01 浏览: 197
好的,我会为您提供一个基于MATLAB的HOG+SVM目标检测案例,包含完整的代码及代码讲解。
首先,让我们来看一下HOG+SVM目标检测的基本流程:
1. 收集和准备训练数据集,包括目标和非目标数据集。
2. 提取HOG特征,将训练数据集转换为HOG特征向量。
3. 使用SVM分类器来训练模型,以分辨目标和非目标。
4. 在测试图像上运行滑动窗口进行目标检测,将每个窗口的HOG特征向量输入到SVM分类器中,以确定窗口是否包含对象。
5. 对于每个被分类为目标的窗口,使用非极大抑制来抑制重叠的窗口,以得到最终的目标检测结果。
接下来,我们将按照上述流程编写代码,并讲解每个步骤的细节。
1. 收集和准备训练数据集
首先,我们需要准备包含目标和非目标数据集的文件夹。在这个案例中,我们使用了INRIA行人数据集,其中包含了正面和负面的图像样本。我们需要将这些图像分别放在两个文件夹中,并将它们的路径保存到.mat文件中。具体代码如下:
```matlab
pos_folder = 'INRIAPerson/train_64x128_H96/pos';
neg_folder = 'INRIAPerson/train_64x128_H96/neg';
pos_img_files = dir(fullfile(pos_folder, '*.png'));
neg_img_files = dir(fullfile(neg_folder, '*.png'));
pos_img_paths = fullfile(pos_folder, {pos_img_files.name}');
neg_img_paths = fullfile(neg_folder, {neg_img_files.name}');
save('img_paths.mat', 'pos_img_paths', 'neg_img_paths');
```
2. 提取HOG特征
接下来,我们需要使用MATLAB内置的HOG函数来提取图像的HOG特征。对于每个图像,我们将其转换为灰度图像,并将其缩放为统一的大小。然后,我们使用HOG函数来提取特征向量,并将其保存到.mat文件中。具体代码如下:
```matlab
% HOG parameters
cell_size = 8;
block_size = 2;
num_bins = 9;
% Load image paths
load('img_paths.mat');
% Extract HOG features for positive samples
pos_features = cell(numel(pos_img_paths), 1);
for i = 1:numel(pos_img_paths)
img = imread(pos_img_paths{i});
img_gray = rgb2gray(img);
img_resized = imresize(img_gray, [128 64]);
pos_features{i} = extractHOGFeatures(img_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
end
% Extract HOG features for negative samples
neg_features = cell(numel(neg_img_paths), 1);
for i = 1:numel(neg_img_paths)
img = imread(neg_img_paths{i});
img_gray = rgb2gray(img);
img_resized = imresize(img_gray, [128 64]);
neg_features{i} = extractHOGFeatures(img_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
end
% Save features to file
save('features.mat', 'pos_features', 'neg_features');
```
3. 使用SVM分类器来训练模型
现在,我们已经提取了所有图像的HOG特征,接下来我们需要使用SVM分类器来训练模型。在这个案例中,我们使用MATLAB内置的fitcsvm函数来训练线性SVM分类器。具体代码如下:
```matlab
% Load features
load('features.mat');
% Combine positive and negative features
X = [vertcat(pos_features{:}); vertcat(neg_features{:})];
Y = [ones(numel(pos_features), 1); zeros(numel(neg_features), 1)];
% Train SVM classifier
svm = fitcsvm(X, Y, 'KernelFunction', 'linear');
% Save SVM model to file
save('svm_model.mat', 'svm');
```
4. 在测试图像上运行滑动窗口进行目标检测
现在,我们已经训练了SVM分类器,接下来我们需要在测试图像上运行滑动窗口进行目标检测。对于每个窗口,我们将其转换为灰度图像,并将其缩放为统一的大小。然后,我们使用HOG函数提取特征向量,并将其输入到SVM分类器中进行分类。如果窗口被分类为目标,我们将其添加到目标集合中。最后,我们使用非极大抑制算法来抑制重叠的窗口,以得到最终的目标检测结果。具体代码如下:
```matlab
% Load SVM model
load('svm_model.mat');
% Load test image
test_img = imread('test.png');
% Sliding window parameters
window_size = [128 64];
step_size = [8 8];
% Initialize variables
target_boxes = [];
target_scores = [];
% Sliding window loop
for r = 1:step_size(1):(size(test_img, 1)-window_size(1))
for c = 1:step_size(2):(size(test_img, 2)-window_size(2))
% Extract window
window = test_img(r:r+window_size(1)-1, c:c+window_size(2)-1, :);
% Convert to grayscale and resize
window_gray = rgb2gray(window);
window_resized = imresize(window_gray, [128 64]);
% Extract HOG features
features = extractHOGFeatures(window_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
% Classify window using SVM
score = predict(svm, features);
% Add to targets if score is positive
if score > 0
target_boxes = [target_boxes; [c r window_size(2) window_size(1)]];
target_scores = [target_scores; score];
end
end
end
% Apply non-maximum suppression
picked_boxes = selectStrongestBbox(target_boxes, target_scores, 'OverlapThreshold', 0.5);
% Draw bounding boxes on test image
figure;
imshow(test_img);
hold on;
for i = 1:size(picked_boxes, 1)
rectangle('Position', picked_boxes(i, :), 'EdgeColor', 'r', 'LineWidth', 2);
end
```
5. 完整代码
最后,让我们将所有代码组合在一起,以获得完整的HOG+SVM目标检测代码。请注意,您需要提前下载INRIA行人数据集,并将其解压到正确的文件夹中。
```matlab
% HOG+SVM Object Detection Example
% By Qishen Huang
%% 1. Collect and prepare training data
% Set folder paths
pos_folder = 'INRIAPerson/train_64x128_H96/pos';
neg_folder = 'INRIAPerson/train_64x128_H96/neg';
% Load positive and negative image paths
pos_img_files = dir(fullfile(pos_folder, '*.png'));
neg_img_files = dir(fullfile(neg_folder, '*.png'));
pos_img_paths = fullfile(pos_folder, {pos_img_files.name}');
neg_img_paths = fullfile(neg_folder, {neg_img_files.name}');
save('img_paths.mat', 'pos_img_paths', 'neg_img_paths');
%% 2. Extract HOG features
% Set HOG parameters
cell_size = 8;
block_size = 2;
num_bins = 9;
% Load image paths
load('img_paths.mat');
% Extract HOG features for positive samples
pos_features = cell(numel(pos_img_paths), 1);
for i = 1:numel(pos_img_paths)
img = imread(pos_img_paths{i});
img_gray = rgb2gray(img);
img_resized = imresize(img_gray, [128 64]);
pos_features{i} = extractHOGFeatures(img_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
end
% Extract HOG features for negative samples
neg_features = cell(numel(neg_img_paths), 1);
for i = 1:numel(neg_img_paths)
img = imread(neg_img_paths{i});
img_gray = rgb2gray(img);
img_resized = imresize(img_gray, [128 64]);
neg_features{i} = extractHOGFeatures(img_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
end
% Save features to file
save('features.mat', 'pos_features', 'neg_features');
%% 3. Train SVM classifier
% Load features
load('features.mat');
% Combine positive and negative features
X = [vertcat(pos_features{:}); vertcat(neg_features{:})];
Y = [ones(numel(pos_features), 1); zeros(numel(neg_features), 1)];
% Train SVM classifier
svm = fitcsvm(X, Y, 'KernelFunction', 'linear');
% Save SVM model to file
save('svm_model.mat', 'svm');
%% 4. Run sliding window on test image
% Load SVM model
load('svm_model.mat');
% Load test image
test_img = imread('test.png');
% Sliding window parameters
window_size = [128 64];
step_size = [8 8];
% Initialize variables
target_boxes = [];
target_scores = [];
% Sliding window loop
for r = 1:step_size(1):(size(test_img, 1)-window_size(1))
for c = 1:step_size(2):(size(test_img, 2)-window_size(2))
% Extract window
window = test_img(r:r+window_size(1)-1, c:c+window_size(2)-1, :);
% Convert to grayscale and resize
window_gray = rgb2gray(window);
window_resized = imresize(window_gray, [128 64]);
% Extract HOG features
features = extractHOGFeatures(window_resized, 'CellSize', [cell_size cell_size],...
'BlockSize', [block_size block_size], 'NumBins', num_bins);
% Classify window using SVM
score = predict(svm, features);
% Add to targets if score is positive
if score > 0
target_boxes = [target_boxes; [c r window_size(2) window_size(1)]];
target_scores = [target_scores; score];
end
end
end
% Apply non-maximum suppression
picked_boxes = selectStrongestBbox(target_boxes, target_scores, 'OverlapThreshold', 0.5);
% Draw bounding boxes on test image
figure;
imshow(test_img);
hold on;
for i = 1:size(picked_boxes, 1)
rectangle('Position', picked_boxes(i, :), 'EdgeColor', 'r', 'LineWidth', 2);
end
```
这就是一个完整的基于MATLAB的HOG+SVM目标检测案例,包含完整的代码及代码讲解。
阅读全文
相关推荐





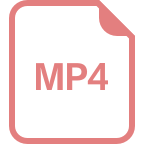








