C#获取当前exe所在文件夹
时间: 2023-08-03 21:04:56 浏览: 115
在C#中获取当前exe所在文件夹可以使用以下代码:
```csharp
string exePath = System.Reflection.Assembly.GetExecutingAssembly().Location;
string exeFolder = System.IO.Path.GetDirectoryName(exePath);
```
第一行代码获取当前运行的exe的路径,第二行代码使用`Path.GetDirectoryName`方法获取该路径所在的文件夹。
相关问题
C#获取exe所在文件夹的路径
在C#中,你可以使用`Assembly.GetExecutingAssembly()`方法获取正在运行的程序的执行文件(.exe),然后结合`DirectoryInfo`类的`DirectoryName`属性来获取该文件所在的目录路径。以下是示例代码:
```csharp
using System;
using System.IO;
using System.Reflection;
class Program
{
static void Main()
{
try
{
// 获取当前执行的程序文件路径
string executingFilePath = Assembly.GetExecutingAssembly().Location;
// 使用Path类的Combine方法确保路径结尾有反斜杠
string directoryPath = Path.GetDirectoryName(executingFilePath);
Console.WriteLine($"The directory of the executable file is: {directoryPath}");
}
catch (Exception ex)
{
Console.WriteLine($"Failed to get the directory: {ex.Message}");
}
}
}
```
这段代码首先获取了`Assembly.GetExecutingAssembly().Location`,得到的是执行文件的完整路径,然后使用`Path.GetDirectoryName()`获取其上级目录即文件夹路径。
unity获取当前项目的文件夹
在Unity引擎中,获取当前项目文件夹(Project Folder)可以使用Unity的内置方法结合路径操作。以下是一个简单的示例:
首先,在Unity编辑器里,你可以通过脚本访问Unity的应用程序目录,然后向下查找ProjectSettings文件夹来获得项目根目录。下面的C#代码片段演示了如何做到这一点:
```csharp
using UnityEngine;
using System.IO;
public class GetCurrentProjectFolder : MonoBehaviour
{
public string projectFolderPath;
void Start()
{
// 获取应用程序目录(即Unity.exe所在的路径)
string applicationDataPath = Application.dataPath;
// 去掉末尾的"/Resources/"或"/StreamingAssets/"
if (applicationDataPath.EndsWith("/Resources/") || applicationDataPath.EndsWith("/StreamingAssets/"))
projectFolderPath = applicationDataPath.Substring(0, applicationDataPath.Length - "/Resources/".Length);
else
projectFolderPath = Path.Combine(applicationDataPath, "../");
Debug.Log("Project folder path: " + projectFolderPath);
}
}
```
这个脚本会在启动游戏时打印出当前项目的完整路径。
**相关问题--:**
1. Unity如何处理跨平台的项目文件路径?
2. 我可以在Unity的哪个菜单选项中找到上述信息?
3. 有没有办法在运行时动态更改项目文件夹路径?
阅读全文
相关推荐
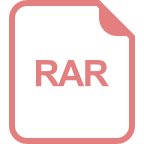

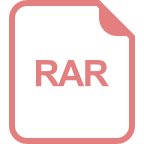



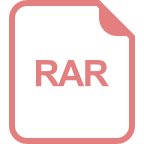





