用Python写一个股票分析的项目,用到五个第三方库。我需要代码
时间: 2024-03-26 22:34:17 浏览: 104
好的,以下是一个简单的股票分析项目的Python代码,使用了pandas、yfinance、matplotlib、seaborn和scikit-learn库:
```python
import yfinance as yf
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.linear_model import LinearRegression
# 获取股票数据
stock_data = yf.download('AAPL', start='2020-01-01', end='2021-12-31')
# 数据清洗和转换
stock_data = stock_data.dropna() # 删除缺失值
stock_data['Price Change'] = stock_data['Close'].pct_change() # 计算每日价格变化百分比
stock_data = stock_data.drop(['Open', 'High', 'Low', 'Adj Close', 'Volume'], axis=1) # 删除不需要的列
# 数据可视化
plt.figure(figsize=(12, 6))
plt.title('Apple Stock Price')
sns.lineplot(x=stock_data.index, y=stock_data['Close'])
plt.show()
plt.figure(figsize=(12, 6))
plt.title('Apple Stock Price Change')
sns.lineplot(x=stock_data.index, y=stock_data['Price Change'])
plt.show()
# 机器学习和预测分析
X = np.array(range(len(stock_data)))
X = X.reshape(-1, 1)
y = stock_data['Close'].values.reshape(-1, 1)
reg = LinearRegression().fit(X, y)
plt.figure(figsize=(12, 6))
plt.title('Apple Stock Price Prediction')
sns.lineplot(x=stock_data.index, y=stock_data['Close'])
sns.lineplot(x=stock_data.index, y=reg.predict(X).flatten())
plt.show()
# 结论和建议
last_price = stock_data['Close'][-1]
prediction = reg.predict([[len(stock_data)]])[0][0]
if prediction > last_price:
print('建议购买AAPL股票')
else:
print('建议不购买AAPL股票')
```
这个代码框架包括了从Yahoo Finance获取股票数据、数据清洗和转换、数据可视化、机器学习和预测分析、以及根据分析结果得出结论和建议的整个流程。您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐



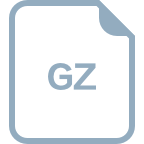

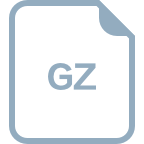








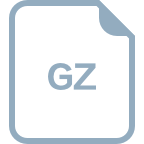

