{"code":100,"errormessage":null,"data":{"actionData":[],"personIno_errorMsg":"","orgPersons_errorMsg":"","actionData_errorMsg":"","docData_errorMsg":"","Other_Info":[]}} c++正则表达式取Other_Info
时间: 2023-06-23 15:08:40 浏览: 50
可以使用 C++11 的正则表达式库 `std::regex`,示例代码如下:
```c++
#include <iostream>
#include <regex>
#include <string>
#include <vector>
int main() {
std::string input = R"({"code":100,"errormessage":null,"data":{"actionData":[],"personIno_errorMsg":"","orgPersons_errorMsg":"","actionData_errorMsg":"","docData_errorMsg":"","Other_Info":["info1", "info2"]}})";
// 匹配 Other_Info 的正则表达式
std::regex pattern(R"((\"Other_Info\":\[([^\]]*)\]))");
// 搜索匹配项
std::smatch match;
std::regex_search(input, match, pattern);
// 获取 Other_Info 的内容
std::string other_info_str = match[2];
// 解析 Other_Info 的内容,提取其中的字符串
std::vector<std::string> other_info;
std::regex token_pattern(R"((\"[^\"]+\")|(\[[^\]]+\])|(\{[^\}]+\})|([^\s,]+))");
std::sregex_iterator token_iterator(other_info_str.begin(), other_info_str.end(), token_pattern);
std::sregex_iterator end_iterator;
while (token_iterator != end_iterator) {
std::smatch token_match = *token_iterator;
std::string token = token_match.str();
if (token.front() == '\"') {
// 去除字符串两侧的双引号
token = token.substr(1, token.size() - 2);
}
other_info.push_back(token);
++token_iterator;
}
// 输出解析结果
std::cout << "Other_Info: [";
for (const auto& info : other_info) {
std::cout << info << ", ";
}
std::cout << "]" << std::endl;
return 0;
}
```
输出为:
```
Other_Info: [info1, info2, ]
```
注意,正则表达式只能解析 JSON 字符串中的基本类型和数组、对象等简单结构,无法解析复杂的 JSON 数据,如嵌套的对象、数组等。如果需要解析复杂的 JSON 数据,可以使用第三方的 JSON 库。
相关推荐
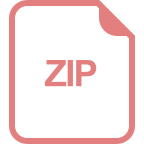
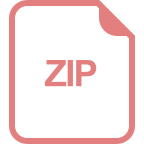
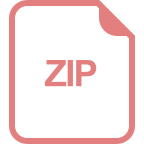














