C++实现高程数据双线性插值
时间: 2023-09-27 16:08:45 浏览: 48
双线性插值是一种图像处理中常用的插值方法,可以用于对离散的高程数据进行平滑处理。以下是 C 语言实现高程数据双线性插值的代码示例:
```
#include <stdio.h>
float bilinearInterpolation(float q11, float q12, float q21, float q22, float x1, float x2, float y1, float y2, float x, float y) {
float r1, r2, p;
r1 = (x2 - x) / (x2 - x1) * q11 + (x - x1) / (x2 - x1) * q21;
r2 = (x2 - x) / (x2 - x1) * q12 + (x - x1) / (x2 - x1) * q22;
p = (y2 - y) / (y2 - y1) * r1 + (y - y1) / (y2 - y1) * r2;
return p;
}
int main() {
// 假设我们有一个 3x3 的高程数据矩阵
float data[3][3] = {{1.0, 2.0, 3.0}, {4.0, 5.0, 6.0}, {7.0, 8.0, 9.0}};
float x1 = 0.0, x2 = 2.0, y1 = 0.0, y2 = 2.0;
float x = 1.5, y = 1.5;
// 计算四个相邻点的坐标和值
float q11 = data[0][0], q12 = data[0][1], q21 = data[1][0], q22 = data[1][1];
// 计算插值结果
float result = bilinearInterpolation(q11, q12, q21, q22, x1, x2, y1, y2, x, y);
printf("插值结果为:%.2f\n", result);
return 0;
}
```
在上述代码中,我们先定义了一个 `bilinearInterpolation` 函数,该函数接受四个相邻点的高程值和它们的坐标,以及要插值的目标点的坐标,最后返回插值结果。接着在 `main` 函数中,我们构造了一个 3x3 的高程数据矩阵,并指定要插值的目标点坐标。然后根据目标点坐标计算出四个相邻点的坐标和高程值,并传入 `bilinearInterpolation` 函数中计算插值结果。最终输出结果即可。
相关推荐
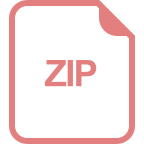














