Dijkstra算法的python编程思想
时间: 2023-08-14 14:32:01 浏览: 93
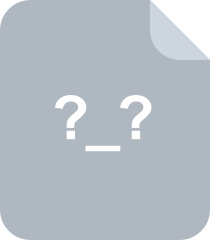
Dijkstra算法python实现
Dijkstra算法是一种用于解决单源最短路径问题的贪心算法,主要基于图的数据结构。它的基本思想是通过不断地扩展当前已经找到的最短路径来找到从源节点到其他所有节点的最短路径。
具体的实现过程可以分为以下几个步骤:
1. 创建一个集合用于存储已经找到最短路径的节点,将源节点添加到集合中,并将源节点的距离设置为0。
2. 创建一个优先队列用于存储未找到最短路径的节点,将所有节点添加到队列中,并将它们的距离设置为正无穷。
3. 从队列中取出距离源节点最近的节点,并将其加入到已找到最短路径的集合中。
4. 遍历当前节点的所有邻居节点,如果当前节点到邻居节点的距离加上当前节点到源节点的距离小于邻居节点的距离,则更新邻居节点的距离。
5. 重复第3步和第4步,直到队列为空或者找到了目标节点。
下面是一个简单的Python实现:
```python
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
current_distance, current_node = heapq.heappop(queue)
if distances[current_node] < current_distance:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
```
其中,`graph`是一个字典类型的变量,用于存储图的邻接表表示方式,`start`是源节点的标识符。函数返回一个字典类型的变量,存储了源节点到其他所有节点的最短距离。
在代码中,我们使用了`heapq`模块来实现优先队列,这样可以保证每次取出的节点都是距离源节点最近的节点。同时,我们使用一个`distances`字典来记录每个节点到源节点的距离,初始值为正无穷。在遍历图的过程中,如果发现新的距离比已有距离更小,就更新距离,并将邻居节点加入到队列中等待处理。
这就是Dijkstra算法的Python编程思想。
阅读全文
相关推荐
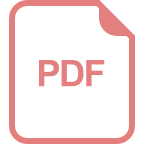
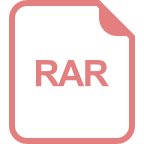
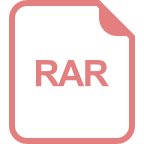
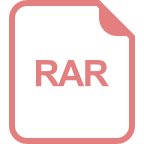
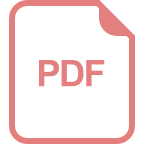
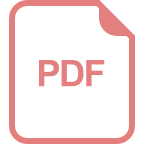
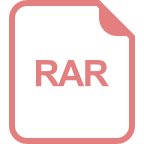
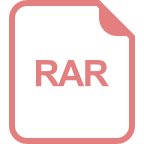
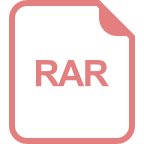
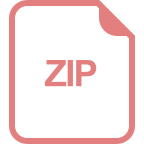
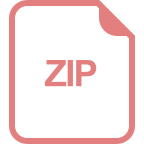
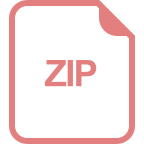
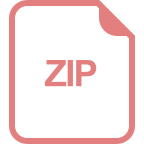
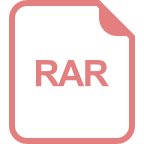
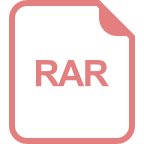
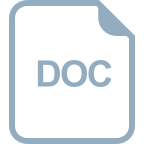