用oc写一个通讯录列表
时间: 2023-12-29 18:51:25 浏览: 46
以下是一个简单的通讯录列表的OC代码实现:
```
// Contact.h
#import <Foundation/Foundation.h>
@interface Contact : NSObject
@property (nonatomic, copy) NSString *name;
@property (nonatomic, copy) NSString *phone;
- (instancetype)initWithName:(NSString *)name phone:(NSString *)phone;
@end
// Contact.m
#import "Contact.h"
@implementation Contact
- (instancetype)initWithName:(NSString *)name phone:(NSString *)phone {
self = [super init];
if (self) {
self.name = name;
self.phone = phone;
}
return self;
}
@end
// ContactCell.h
#import <UIKit/UIKit.h>
@interface ContactCell : UITableViewCell
@property (nonatomic, strong) UILabel *nameLabel;
@property (nonatomic, strong) UILabel *phoneLabel;
@end
// ContactCell.m
#import "ContactCell.h"
@implementation ContactCell
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier {
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier];
if (self) {
self.nameLabel = [[UILabel alloc] initWithFrame:CGRectMake(15, 10, 200, 20)];
[self.contentView addSubview:self.nameLabel];
self.phoneLabel = [[UILabel alloc] initWithFrame:CGRectMake(15, 35, 200, 20)];
[self.contentView addSubview:self.phoneLabel];
}
return self;
}
@end
// ContactListViewController.h
#import <UIKit/UIKit.h>
@interface ContactListViewController : UITableViewController
@end
// ContactListViewController.m
#import "ContactListViewController.h"
#import "Contact.h"
#import "ContactCell.h"
@interface ContactListViewController ()
@property (nonatomic, strong) NSMutableArray<Contact *> *contacts;
@end
@implementation ContactListViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.navigationItem.title = @"Contacts";
UIBarButtonItem *addButton = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemAdd target:self action:@selector(addContact)];
self.navigationItem.rightBarButtonItem = addButton;
self.contacts = [NSMutableArray array];
Contact *contact1 = [[Contact alloc] initWithName:@"John Smith" phone:@"555-1234"];
[self.contacts addObject:contact1];
Contact *contact2 = [[Contact alloc] initWithName:@"Jane Doe" phone:@"555-5678"];
[self.contacts addObject:contact2];
[self.tableView registerClass:[ContactCell class] forCellReuseIdentifier:@"ContactCell"];
}
#pragma mark - Table view data source
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.contacts.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
ContactCell *cell = [tableView dequeueReusableCellWithIdentifier:@"ContactCell" forIndexPath:indexPath];
Contact *contact = self.contacts[indexPath.row];
cell.nameLabel.text = contact.name;
cell.phoneLabel.text = contact.phone;
return cell;
}
#pragma mark - Actions
- (void)addContact {
UIAlertController *alert = [UIAlertController alertControllerWithTitle:@"Add Contact" message:nil preferredStyle:UIAlertControllerStyleAlert];
[alert addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) {
textField.placeholder = @"Name";
}];
[alert addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) {
textField.placeholder = @"Phone";
}];
UIAlertAction *addAction = [UIAlertAction actionWithTitle:@"Add" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) {
UITextField *nameField = alert.textFields[0];
UITextField *phoneField = alert.textFields[1];
Contact *contact = [[Contact alloc] initWithName:nameField.text phone:phoneField.text];
[self.contacts addObject:contact];
[self.tableView reloadData];
}];
UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"Cancel" style:UIAlertActionStyleCancel handler:nil];
[alert addAction:addAction];
[alert addAction:cancelAction];
[self presentViewController:alert animated:YES completion:nil];
}
@end
```
在这个实现中,我们创建了一个`Contact`类来表示每个联系人,一个`ContactCell`类来自定义通讯录列表中的单元格,一个`ContactListViewController`类来管理通讯录列表。我们使用`NSMutableArray`来存储所有的联系人,并在视图加载时向其中添加了一些示例联系人。
在`ContactListViewController`中,我们实现了`UITableViewDataSource`协议和`UITableViewDelegate`协议中的方法来显示通讯录列表和处理用户添加新联系人的操作。我们还添加了一个`UIBarButtonItem`,当用户点击它时会出现一个`UIAlertController`来允许用户输入新联系人的信息。最后,我们在`viewDidLoad`方法中注册了`ContactCell`类以供表视图使用。
这只是一个简单的例子,你可以根据自己的需要对其进行修改和扩展。
相关推荐
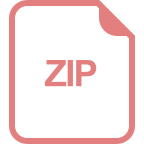














