用Pyhon写一个关于维护仪器仪表的代码
时间: 2024-03-17 16:39:51 浏览: 153
以下是一个简单的用Python编写的维护仪器仪表的示例代码:
```python
class Instrument:
def __init__(self, name, location, inspection_period, maintenance_period):
self.name = name # 仪器名称
self.location = location # 安装位置
self.inspection_period = inspection_period # 检定周期
self.maintenance_period = maintenance_period # 保养周期
self.last_inspection_date = '' # 上次检定日期
self.last_maintenance_date = '' # 上次保养日期
def inspection(self, inspection_date):
self.last_inspection_date = inspection_date
print(f'{self.name}检定完成')
def maintenance(self, maintenance_date):
self.last_maintenance_date = maintenance_date
print(f'{self.name}保养完成')
def check_inspection(self, today_date):
if self.last_inspection_date == '':
return False
else:
days = (today_date - self.last_inspection_date).days
if days >= self.inspection_period:
return True
else:
return False
def check_maintenance(self, today_date):
if self.last_maintenance_date == '':
return False
else:
days = (today_date - self.last_maintenance_date).days
if days >= self.maintenance_period:
return True
else:
return False
# 测试代码
instrument1 = Instrument('仪器1', '位置1', 365, 180)
instrument2 = Instrument('仪器2', '位置2', 180, 90)
today_date = datetime.date.today()
print(today_date)
if instrument1.check_inspection(today_date):
instrument1.inspection(today_date)
if instrument1.check_maintenance(today_date):
instrument1.maintenance(today_date)
if instrument2.check_inspection(today_date):
instrument2.inspection(today_date)
if instrument2.check_maintenance(today_date):
instrument2.maintenance(today_date)
```
以上代码定义了一个Instrument类,包括仪器的名称、安装位置、检定周期、保养周期、上次检定日期、上次保养日期等基本信息。类中定义了inspection()方法用于进行检定,maintenance()方法用于进行保养,check_inspection()方法用于检查是否需要进行检定,check_maintenance()方法用于检查是否需要进行保养。代码中还包括了一些测试代码,用于测试仪器是否需要进行检定和保养,并进行相应的操作。
阅读全文
相关推荐




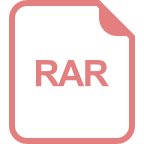












