python 中能源指数和工业产值脉冲响应函数的运用附有代码
时间: 2023-12-03 19:46:56 浏览: 84
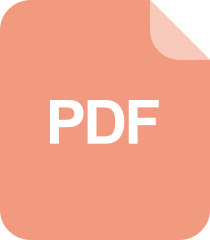
Python应用实现双指数函数及拟合代码实例
能源指数和工业产值脉冲响应函数是宏观经济学中常用的分析工具,可以用来研究经济的波动和变化。在 Python 中,我们可以使用 pandas 和 statsmodels 库来计算和绘制这些指标和函数。
首先,需要准备一些数据。以下是一个示例数据集,包含了工业产值和能源消费的时间序列数据:
```
import pandas as pd
# 创建一个示例数据集
data = {'GDP': [100, 110, 120, 130, 140, 150, 160, 170, 180, 190, 200],
'Energy': [50, 60, 70, 80, 90, 100, 110, 120, 130, 140, 150]}
df = pd.DataFrame(data, index=pd.period_range('2010-01', periods=11, freq='M'))
```
接下来,我们可以使用 pandas 的 pct_change() 函数计算出每个月的增长率,然后使用 cumsum() 函数计算出每个月的累计增长率。
```
# 计算增长率和累计增长率
gdp_growth = df['GDP'].pct_change().cumsum()
energy_growth = df['Energy'].pct_change().cumsum()
```
现在,我们可以绘制出工业产值和能源消费的时间序列图和增长率图:
```
import matplotlib.pyplot as plt
# 绘制时间序列图
fig, ax = plt.subplots()
df.plot(ax=ax)
ax.set_ylabel('Billions of dollars / Millions of BTUs')
plt.show()
# 绘制增长率图
fig, ax = plt.subplots()
gdp_growth.plot(ax=ax, label='GDP')
energy_growth.plot(ax=ax, label='Energy')
ax.legend()
ax.set_ylabel('Cumulative growth rate')
plt.show()
```
接下来,我们可以使用 statsmodels 库中的 impulse_response() 函数计算脉冲响应函数。脉冲响应函数可以用来研究一个变量对另一个变量的影响,例如工业产值对能源消费的影响。
```
import statsmodels.api as sm
# 计算工业产值对能源消费的脉冲响应函数
model = sm.tsa.VAR(df)
irf = model.irf(10)
irf.plot(impulse='GDP', response='Energy')
plt.show()
```
以上代码将计算工业产值对能源消费的脉冲响应函数,并绘制出结果。我们可以看到,工业产值的增加会导致对能源消费的增加,但是这种影响是逐渐消失的。
完整的代码如下:
```
import pandas as pd
import matplotlib.pyplot as plt
import statsmodels.api as sm
# 创建一个示例数据集
data = {'GDP': [100, 110, 120, 130, 140, 150, 160, 170, 180, 190, 200],
'Energy': [50, 60, 70, 80, 90, 100, 110, 120, 130, 140, 150]}
df = pd.DataFrame(data, index=pd.period_range('2010-01', periods=11, freq='M'))
# 计算增长率和累计增长率
gdp_growth = df['GDP'].pct_change().cumsum()
energy_growth = df['Energy'].pct_change().cumsum()
# 绘制时间序列图
fig, ax = plt.subplots()
df.plot(ax=ax)
ax.set_ylabel('Billions of dollars / Millions of BTUs')
plt.show()
# 绘制增长率图
fig, ax = plt.subplots()
gdp_growth.plot(ax=ax, label='GDP')
energy_growth.plot(ax=ax, label='Energy')
ax.legend()
ax.set_ylabel('Cumulative growth rate')
plt.show()
# 计算工业产值对能源消费的脉冲响应函数
model = sm.tsa.VAR(df)
irf = model.irf(10)
irf.plot(impulse='GDP', response='Energy')
plt.show()
```
阅读全文
相关推荐
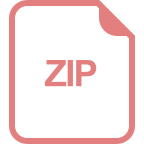
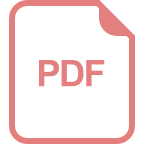
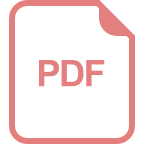
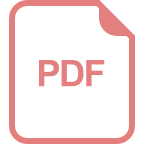
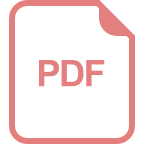
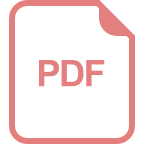
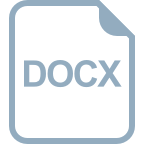
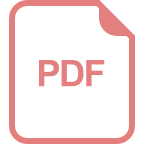
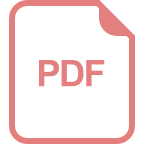
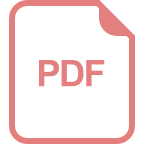
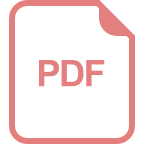
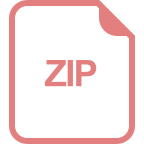
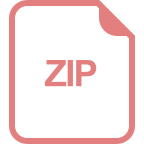
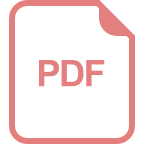
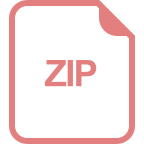
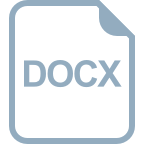