用java写一个多线程实现银行存取款系统并且写两名顾客,每人分三次、每次100元将钱汇入count。 每进行一次汇款,便输出汇款人和账户总额。
时间: 2024-02-21 18:59:08 浏览: 69
下面是一个使用Java多线程实现银行存取款系统并且包括两名顾客的示例代码:
```java
public class BankAccount {
private int balance;
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public synchronized void deposit(int amount) {
balance += amount;
System.out.println("Deposited " + amount + "; balance is now " + balance);
}
public synchronized void withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
System.out.println("Withdrew " + amount + "; balance is now " + balance);
} else {
System.out.println("Insufficient funds");
}
}
public int getBalance() {
return balance;
}
}
public class BankTransaction implements Runnable {
private BankAccount account;
private int amount;
private boolean deposit;
private String customerName;
public BankTransaction(BankAccount account, int amount, boolean deposit, String customerName) {
this.account = account;
this.amount = amount;
this.deposit = deposit;
this.customerName = customerName;
}
public void run() {
if (deposit) {
account.deposit(amount);
} else {
account.withdraw(amount);
}
System.out.println(customerName + " balance is now " + account.getBalance());
}
}
public class BankDemo {
public static void main(String[] args) {
BankAccount account = new BankAccount(0);
Thread t1 = new Thread(new BankTransaction(account, 100, true, "Customer 1"));
Thread t2 = new Thread(new BankTransaction(account, 100, true, "Customer 2"));
Thread t3 = new Thread(new BankTransaction(account, 100, true, "Customer 1"));
Thread t4 = new Thread(new BankTransaction(account, 100, true, "Customer 2"));
Thread t5 = new Thread(new BankTransaction(account, 100, true, "Customer 1"));
Thread t6 = new Thread(new BankTransaction(account, 100, true, "Customer 2"));
t1.start();
t2.start();
t3.start();
t4.start();
t5.start();
t6.start();
}
}
```
该示例中,`BankAccount` 类表示银行账户,包含一个账户余额变量和 `deposit` 和 `withdraw` 方法用于存款和取款。这两个方法都使用 `synchronized` 关键字来确保在多线程环境下的安全性。
`BankTransaction` 类实现了 `Runnable` 接口,表示银行交易,其中包含一个银行账户、一个交易金额、一个布尔值表示是否为存款,以及一个字符串表示顾客姓名。`run` 方法根据布尔值调用 `deposit` 或 `withdraw` 方法,并在执行完交易后输出顾客姓名和账户余额。
`BankDemo` 类用于演示多线程银行存取款系统,并包括两名顾客。在主函数中创建一个 `BankAccount` 对象和六个 `BankTransaction` 对象,每个顾客分别进行三次存款,每次存款 100 元。在每次存款后,输出顾客姓名和账户余额。在运行过程中,`BankAccount` 的 `deposit` 和 `withdraw` 方法将确保多线程环境下的数据安全。
阅读全文
相关推荐






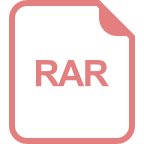
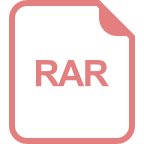
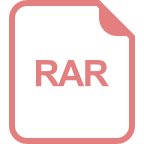
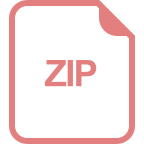
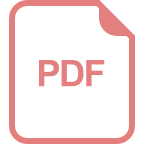
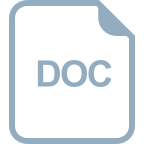

