arduino ticker库写数码管扫描
时间: 2023-07-16 13:11:48 浏览: 116
使用 Arduino Ticker 库和数码管模块,可以实现数码管的扫描显示。以下是一个示例代码,可以实现四位数码管的依次扫描显示。
首先,需要引入 Ticker 库和数码管库:
```
#include <Ticker.h>
#include <TM1637Display.h>
```
然后,定义数码管的引脚和 Ticker 对象:
```
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
Ticker ticker;
```
接着,定义一个计数器变量 count,以及一个回调函数 tickerCallback,该回调函数在 Ticker 触发时会执行。在回调函数中,将计数器 count 的值转换为字符串,然后将其显示在数码管上:
```
int count = 0;
void tickerCallback() {
String countStr = String(count, DEC);
while (countStr.length() < 4) {
countStr = " " + countStr;
}
display.showNumberDec(atoi(countStr.c_str()));
count++;
if (count > 9999) {
count = 0;
}
}
```
最后,在 setup() 函数中初始化数码管和 Ticker 对象,并开始 Ticker:
```
void setup() {
display.setBrightness(0x0f);
ticker.attach_ms(10, tickerCallback);
}
```
完整代码如下:
```
#include <Ticker.h>
#include <TM1637Display.h>
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
Ticker ticker;
int count = 0;
void tickerCallback() {
String countStr = String(count, DEC);
while (countStr.length() < 4) {
countStr = " " + countStr;
}
display.showNumberDec(atoi(countStr.c_str()));
count++;
if (count > 9999) {
count = 0;
}
}
void setup() {
display.setBrightness(0x0f);
ticker.attach_ms(10, tickerCallback);
}
void loop() {
// do nothing
}
```
在上述代码中,我们将数码管的亮度设置为最大值(0x0f),并将 Ticker 的间隔设置为 10 毫秒。在回调函数中,我们将计数器 count 的值转换为四位数的字符串,并将其显示在数码管上。每次触发 Ticker,计数器 count 就加一。如果计数器超过了 9999,就将其重置为 0。
该代码可以实现四位数码管的扫描显示,每个数字显示 10 毫秒。您可以根据需要,调整 Ticker 的间隔和数码管的亮度等参数。
阅读全文
相关推荐











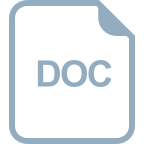
