假定一个文本文件中包含未指定个数的考试分数,请编写程序实现以下操作。(1)程序运行时应该提示用户输入一个文件名,并将分数写入到文件中,文件中的分数用空格分隔;(2)从文件中读取分数,以列表的方式打印输出所有的分数,同时计算分数之和、平均数以及最大分数;(3)将分数之和、平均数以及最大分数追加到文件中。
时间: 2024-03-17 11:43:15 浏览: 18
下面是实现题目要求的 Python 代码:
```python
# 从文件中读取分数,返回分数列表
def read_scores(filename):
scores = []
with open(filename, 'r') as f:
for line in f:
scores += [int(x) for x in line.strip().split()]
return scores
# 将分数写入文件
def write_scores(filename, scores):
with open(filename, 'w') as f:
for score in scores:
f.write(str(score) + ' ')
# 计算分数之和、平均数以及最大分数
def calculate_scores(scores):
if not scores:
return 0, 0, 0
total = sum(scores)
average = total / len(scores)
maximum = max(scores)
return total, average, maximum
# 将分数统计结果追加到文件中
def append_statistics(filename, total, average, maximum):
with open(filename, 'a') as f:
f.write('\n')
f.write(f'Total: {total}\n')
f.write(f'Average: {average:.2f}\n')
f.write(f'Maximum: {maximum}\n')
if __name__ == '__main__':
# 从用户输入中获取文件名
filename = input('Please enter a filename: ')
# 从文件中读取分数
scores = read_scores(filename)
# 打印分数列表、分数之和、平均数以及最大分数
print('Scores:', scores)
total, average, maximum = calculate_scores(scores)
print('Total:', total)
print('Average:', average)
print('Maximum:', maximum)
# 将分数之和、平均数以及最大分数追加到文件中
append_statistics(filename, total, average, maximum)
```
代码中的 `read_scores()` 函数从文件中读取分数,并将分数存储在一个列表中返回;`write_scores()` 函数将分数列表写入文件;`calculate_scores()` 函数计算分数之和、平均数以及最大分数,并将结果作为元组返回;`append_statistics()` 函数将分数统计结果追加到文件中。主程序中,我们先从用户输入中获取文件名,然后依次调用上述函数实现题目要求的功能。
相关推荐
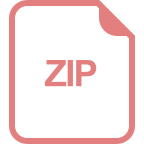
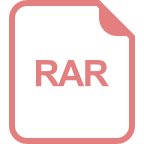














