使用go语言生成字符画
时间: 2023-07-07 08:33:12 浏览: 47
使用Go语言生成字符画可以使用image包和color包。下面是一个示例程序,可以将任意一张图片转换成字符画并输出到控制台:
```
package main
import (
"fmt"
"image"
"image/color"
"image/jpeg"
"os"
)
func main() {
// 打开图片文件
file, err := os.Open("test.jpg")
if err != nil {
fmt.Println(err)
return
}
defer file.Close()
// 解码图片
img, _, err := image.Decode(file)
if err != nil {
fmt.Println(err)
return
}
// 缩小图片尺寸
img = resize(img, 200)
// 转换成字符画
ascii := toAscii(img)
// 输出字符画到控制台
fmt.Println(ascii)
}
// 缩小图片尺寸
func resize(img image.Image, size int) image.Image {
bounds := img.Bounds()
ratio := bounds.Dx() / size
newBounds := bounds
newBounds.Max.X /= ratio
newBounds.Max.Y /= ratio
newImg := image.NewRGBA(newBounds)
for x := 0; x < newBounds.Max.X; x++ {
for y := 0; y < newBounds.Max.Y; y++ {
color := img.At(x*ratio, y*ratio)
newImg.Set(x, y, color)
}
}
return newImg
}
// 转换成字符画
func toAscii(img image.Image) string {
ascii := ""
for y := 0; y < img.Bounds().Max.Y; y++ {
for x := 0; x < img.Bounds().Max.X; x++ {
color := img.At(x, y)
gray := colorToGray(color)
ascii += grayToChar(gray)
}
ascii += "\n"
}
return ascii
}
// 将颜色转换成灰度值
func colorToGray(color color.Color) uint8 {
r, g, b, _ := color.RGBA()
return uint8((19595*r + 38469*g + 7472*b) >> 16)
}
// 将灰度值转换成字符
func grayToChar(gray uint8) string {
chars := "@#S%?*+;:,."
index := int(gray) * (len(chars) - 1) / 255
return string(chars[index])
}
```
需要注意的是,这个程序只能在控制台中输出字符画,如果需要保存到文件中,需要使用文件输出函数。另外,由于字符画的尺寸比原图小很多,输出的字符画可能会比较模糊,可以根据需要调整缩小尺寸的比例来改善效果。
相关推荐
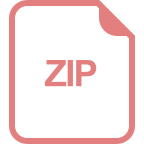
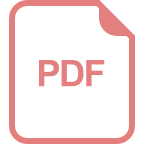
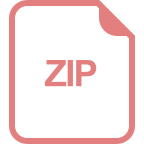














