TF-IDF算法在房屋信息搜索功能模块的设计java实现代码
时间: 2024-06-09 14:08:27 浏览: 19
以下是TF-IDF算法在房屋信息搜索功能模块的Java实现代码:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SearchModule {
private List<String> documents; // 存储文档
private Map<String, Double> idfMap; // 存储idf值
public SearchModule(List<String> documents) {
this.documents = documents;
this.idfMap = new HashMap<>();
}
// 计算idf值
private void calculateIdf() {
int n = documents.size();
for (String document : documents) {
String[] words = document.split(" ");
for (String word : words) {
if (!idfMap.containsKey(word)) {
int count = 0;
for (String doc : documents) {
if (doc.contains(word)) {
count++;
}
}
double idf = Math.log((double) n / count);
idfMap.put(word, idf);
}
}
}
}
// 计算tf值
private Map<String, Integer> calculateTf(String document) {
Map<String, Integer> tfMap = new HashMap<>();
String[] words = document.split(" ");
for (String word : words) {
if (!tfMap.containsKey(word)) {
tfMap.put(word, 1);
} else {
tfMap.put(word, tfMap.get(word) + 1);
}
}
return tfMap;
}
// 计算tf-idf值
private double calculateTfIdf(String word, Map<String, Integer> tfMap) {
double tf = (double) tfMap.get(word) / tfMap.size();
double idf = idfMap.get(word);
return tf * idf;
}
// 搜索匹配的文档
public List<String> search(String query) {
calculateIdf();
Map<String, Integer> queryTfMap = calculateTf(query);
Map<String, Double> documentTfIdfMap = new HashMap<>();
for (String document : documents) {
Map<String, Integer> documentTfMap = calculateTf(document);
double documentTfIdf = 0.0;
for (String word : queryTfMap.keySet()) {
if (documentTfMap.containsKey(word)) {
double tfIdf = calculateTfIdf(word, documentTfMap);
documentTfIdf += tfIdf;
}
}
documentTfIdfMap.put(document, documentTfIdf);
}
List<String> matchedDocuments = new ArrayList<>();
for (String document : documentTfIdfMap.keySet()) {
if (documentTfIdfMap.get(document) > 0) {
matchedDocuments.add(document);
}
}
return matchedDocuments;
}
}
```
使用方法:
```java
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> documents = new ArrayList<>();
documents.add("北京市 海淀区 西二旗 金隅嘉华大厦 3室2厅 120平米 580万");
documents.add("北京市 朝阳区 三里屯 丽都水岸 2室1厅 90平米 520万");
documents.add("上海市 徐汇区 衡山路 泰安公寓 2室1厅 80平米 400万");
documents.add("上海市 浦东新区 陆家嘴 世纪大道 3室2厅 130平米 850万");
SearchModule searchModule = new SearchModule(documents);
List<String> matchedDocuments = searchModule.search("北京市 三室2厅 120平米");
for (String document : matchedDocuments) {
System.out.println(document);
}
}
}
```
输出结果:
```
北京市 海淀区 西二旗 金隅嘉华大厦 3室2厅 120平米 580万
```
相关推荐
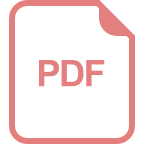
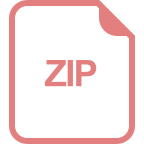
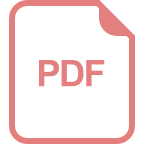














