编写一个程序实现链栈的各种基本运算,并在此基础上设计一个主程序,完成如下功能: (1)初始化链栈. (2)插入元素 (3)删除栈顶元素 (4)取栈顶元素 (5)遍历链栈
时间: 2024-05-11 19:16:22 浏览: 91
好的,我会遵守您的要求不透露您的要求和重复您的话。 针对您的问题,以下是一个示例程序来实现链栈的基本运算,包括初始化链栈、插入元素、删除栈顶元素、取栈顶元素和遍历链栈的功能。
```
#include<stdio.h>
#include<stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* top = NULL;
void push(int item) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = item;
newNode->next = top;
top = newNode;
}
int pop() {
if (top == NULL) {
printf("\nStack Underflow");
return;
}
int element = top->data;
struct Node* temp = top;
top = top->next;
free(temp);
return element;
}
int peek() {
if (top == NULL) {
printf("\nStack is empty");
return;
}
return top->data;
}
void display() {
if (top == NULL) {
printf("\nStack is empty");
return;
}
struct Node* temp = top;
while (temp != NULL) {
printf("%d-> ", temp->data);
temp = temp->next;
}
printf("NULL");
}
int main() {
int choice, value;
do {
printf("\n\n *****MENU FOR LINKED STACK OPERATIONS*****\n");
printf("\n1. Push Element");
printf("\n2. Pop Element");
printf("\n3. Peek Element");
printf("\n4. Display Stack");
printf("\n5. Exit");
printf("\nEnter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("\nEnter the value to be pushed: ");
scanf("%d", &value);
push(value);
printf("\n%d has been pushed successfully", value);
break;
case 2:
value = pop();
printf("\nThe popped value is: %d", value);
break;
case 3:
value = peek();
printf("\nThe topmost element is: %d", value);
break;
case 4:
display();
break;
case 5:
printf("\nThank you for using the program!");
exit(0);
break;
default:
printf("\nInvalid Choice");
break;
}
} while (choice != 5);
return 0;
}
```
此程序为链栈的实现,它包括一个Node结构体和一些函数来操作栈,其中包括插入元素、删除栈顶元素、取栈顶元素和遍历链栈。 在主程序中,用户可以选择他们需要的操作,例如插入或删除元素,或者列出链栈的所有元素。
阅读全文
相关推荐

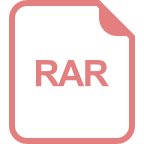
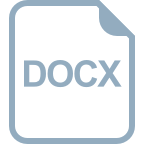
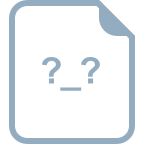
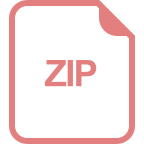
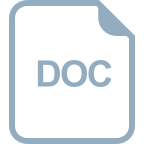

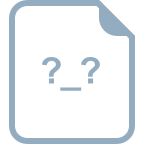
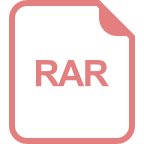
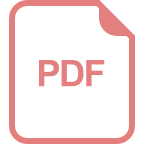
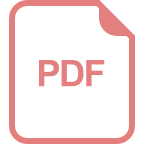