#include <bits/stdc++.h> using namespace std; int R[16], G[16], B[16]; int main() { for (int i = 0; i < 16; i++) { cin >> R[i] >> G[i] >> B[i]; } int a, b, c; while (cin >> a >> b >> c && a != -1) { int Min = (R[0] - a) * (R[0] - a) + (G[0] - b) * (G[0] - b) + (B[0] - c) * (B[0] - c); int d = R[0], e = G[0], f = B[0]; for (int i = 1; i < 16; i++) { if (Min > (R[i] - a) * (R[i] - a) + (G[i] - b) * (G[i] - b) + (B[i] - c) * (B[i] - c)) { Min = (R[i] - a) * (R[i] - a) + (G[i] - b) * (G[i] - b) + (B[i] - c) * (B[i] - c); d = R[i], e = G[i], f = B[i]; } } cout << '(' << a << ',' << b << ',' << c << ')' << " maps to " << '(' << d << ',' << e << ',' << f << ')' << endl; } return 0; } 请把这个代码用优先队列实现
时间: 2024-03-16 21:43:47 浏览: 23
这个代码可以使用优先队列来实现。具体来说,我们可以将所有颜色点按照它们与目标点的距离从小到大排序,然后取出距离最小的点作为最终的映射。
以下是使用优先队列实现的样例代码:
```c++
#include <iostream>
#include <queue>
#include <cmath>
using namespace std;
struct Color {
int r, g, b;
double dist;
bool operator>(const Color& other) const { // 重载运算符
return dist > other.dist;
}
};
int main() {
vector<Color> colors;
for (int i = 0; i < 16; i++) {
int r, g, b;
cin >> r >> g >> b;
colors.push_back({r, g, b, 0});
}
int a, b, c;
while (cin >> a >> b >> c && a != -1) {
// 计算每个颜色点与目标点的距离
for (int i = 0; i < 16; i++) {
double r = colors[i].r - a;
double g = colors[i].g - b;
double b = colors[i].b - c;
colors[i].dist = sqrt(r * r + g * g + b * b);
}
// 使用小根堆来存储颜色点,按照距离从小到大排序
priority_queue<Color, vector<Color>, greater<Color>> pq;
for (int i = 0; i < 16; i++) {
pq.push(colors[i]);
}
// 取出距离最小的点作为最终的映射
Color closest = pq.top();
cout << '(' << a << ',' << b << ',' << c << ')' << " maps to "
<< '(' << closest.r << ',' << closest.g << ',' << closest.b << ')' << endl;
}
return 0;
}
```
在这个代码中,我们定义了一个结构体 `Color`,用来存储颜色点的 RGB 值和与目标点的距离。为了方便使用优先队列,我们重载了运算符 `>`,使得颜色点可以按照距离从小到大排序。
在主函数中,我们首先读入所有颜色点,然后对于每个目标点,计算所有颜色点与它的距离,并将它们放入一个小根堆中。接着,我们取出堆顶元素,即距离最小的颜色点,作为最终的映射。
需要注意的是,由于距离的计算涉及到浮点数运算,所以我们需要使用 `sqrt` 函数来计算距离的平方根。另外,由于题目中给出的颜色点数量较小,所以我们可以直接将所有颜色点存储在一个向量中,而不必使用动态数组或链表。
相关推荐
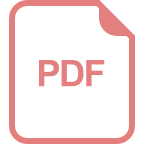
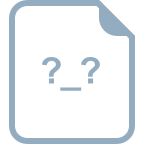
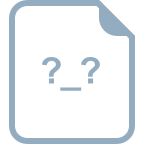
















