HTML打地鼠小游戏
时间: 2023-07-09 10:15:01 浏览: 162
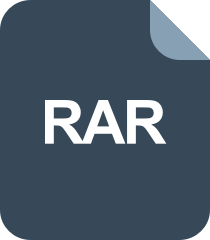
HTML5打地鼠小游戏
好的,HTML打地鼠小游戏可以通过JavaScript来实现。以下是一个简单的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠小游戏</title>
<style>
.container {
display: flex;
flex-wrap: wrap;
width: 300px;
height: 300px;
border: 1px solid #000;
padding: 10px;
}
.hole {
width: 90px;
height: 90px;
background-color: #ccc;
border: 1px solid #000;
text-align: center;
line-height: 90px;
font-size: 30px;
cursor: pointer;
margin: 5px;
}
.hole.active {
background-color: #ff6b6b;
color: #fff;
}
</style>
</head>
<body>
<div class="container"></div>
<script>
const holes = document.querySelectorAll('.hole');
let score = 0;
let lastHole;
function randomTime(min, max) {
return Math.round(Math.random() * (max - min) + min);
}
function randomHole(holes) {
const idx = Math.floor(Math.random() * holes.length);
const hole = holes[idx];
if (hole === lastHole) {
return randomHole(holes);
}
lastHole = hole;
return hole;
}
function peep() {
const time = randomTime(200, 1000);
const hole = randomHole(holes);
hole.classList.add('active');
setTimeout(() => {
hole.classList.remove('active');
if (!timeUp) peep();
}, time);
}
function startGame() {
score = 0;
timeUp = false;
scoreBoard.textContent = 0;
peep();
setTimeout(() => timeUp = true, 10000);
}
function bonk(e) {
if (!e.isTrusted) return;
score++;
this.classList.remove('active');
scoreBoard.textContent = score;
}
holes.forEach(hole => hole.addEventListener('click', bonk));
</script>
</body>
</html>
```
这个小游戏的实现思路如下:
- 通过 CSS 布局和样式创建一个 3 x 3 的打地鼠区域。
- 使用 JavaScript 选择所有的打地鼠洞,以及记录分数和最后一个洞口。
- 随机生成一个时间,随机选择一个洞口,将该洞口设置为“active”状态。
- 点击一个洞口时,如果它处于“active”状态,分数加一,该洞口取消“active”状态。
- 在规定的时间内重复上述步骤,最后结束游戏。
希望这个示例代码能够帮助你了解如何使用 HTML 和 JavaScript 创建打地鼠小游戏。
阅读全文
相关推荐
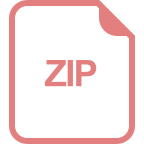
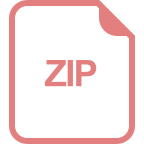















