举一个移动one net 与esp8266 mqtt通信的例子,要有例程与数据格式说明
时间: 2024-03-05 11:47:33 浏览: 96
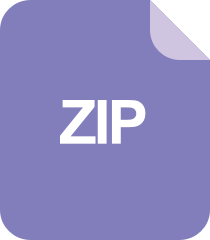
MQTT 1路开关控制(WiFi例程)_STM32+esp8266_stm32wifionenet_onenet_mqttstm

好的,下面是基于ESP8266模块和移动OneNet平台的MQTT协议进行通信的一个例子,包括了数据格式和具体的示例代码:
1. 数据格式
设备上传数据时,需要将数据封装成JSON格式,并发布到特定的主题中。OneNet平台会对上传的数据进行解析和转发。
设备上传数据的主题格式为:'$dp' + '/sys/' + productId + '/' + deviceKey + '/thing/event/property/post',其中productId为产品ID,deviceKey为设备API-key。
JSON格式的数据示例:
```
{
"id":"12345",
"params":{
"temperature":25.5,
"humidity":50.0
},
"method":"thing.event.property.post"
}
```
其中,id为消息ID,params为设备属性信息,method为消息方法。
2. 示例代码
下面是一个基于ESP8266模块和移动OneNet平台的MQTT协议进行通信的示例代码。代码中使用了ESP8266WiFi库和PubSubClient库,需要提前安装。
```c++
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
// 替换为你的WiFi名称和密码
const char* ssid = "your_ssid";
const char* password = "your_password";
// 替换为你的设备API-key和产品ID
const char* deviceKey = "your_device_api_key";
const char* productId = "your_product_id";
// 替换为你的OneNet平台MQTT服务器地址和端口
const char* mqttServer = "mqtt.heclouds.com";
int mqttPort = 6002;
WiFiClient wifiClient;
PubSubClient mqttClient(wifiClient);
void setup() {
// 初始化串口连接
Serial.begin(9600);
// 连接WiFi网络
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// 连接OneNet平台的MQTT服务器
mqttClient.setServer(mqttServer, mqttPort);
while (!mqttClient.connected()) {
String clientId = "esp8266-" + String(random(0xffff), HEX);
if (mqttClient.connect(clientId.c_str(), deviceKey, NULL)) {
Serial.println("Connected to MQTT server");
} else {
Serial.println("Failed to connect to MQTT server");
delay(1000);
}
}
// 订阅主题
String topic = String("$dp/sys/") + productId + "/" + deviceKey + "/thing/event/property/post";
mqttClient.subscribe(topic.c_str());
}
void loop() {
// 模拟上传温湿度数据
float temperature = random(10, 30) + 0.5;
float humidity = random(30, 80) + 0.5;
// 将数据封装成JSON格式
String payload = String("{\"id\":\"") + String(random(0xffff), HEX) + "\",\"params\":{\"temperature\":" + String(temperature) + ",\"humidity\":" + String(humidity) + "},\"method\":\"thing.event.property.post\"}";
// 将数据发布到OneNet平台的MQTT服务器
String topic = String("$dp/sys/") + productId + "/" + deviceKey + "/thing/event/property/post";
mqttClient.publish(topic.c_str(), payload.c_str());
// 等待3秒后再上传数据
delay(3000);
}
```
在这个示例代码中,ESP8266模块每隔3秒钟上传一次温湿度数据到OneNet平台。需要替换代码中的WiFi名称、密码、设备API-key、产品ID和MQTT服务器地址和端口等信息,才能正常运行。
同时,需要注意的是,在使用OneNet平台的MQTT协议进行通信时,需要进行认证和鉴权,避免数据泄露和安全问题。
阅读全文
相关推荐
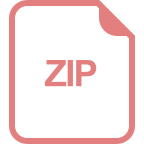
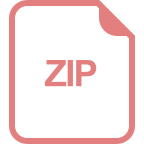
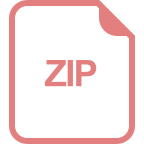
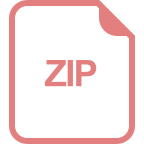
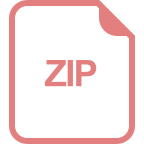
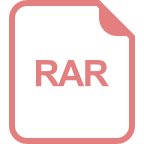
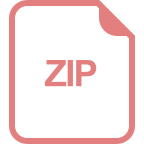
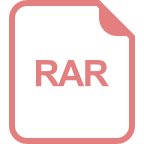
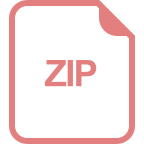
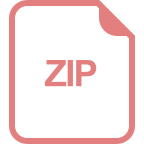
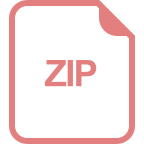
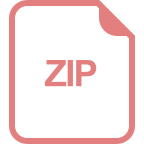
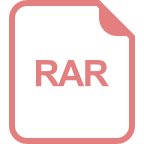