一、请在VS2013中编写代码,定义一个员工工资的结构体类型,成员包括职工号(ID)、工资(salary)和指针变量(next),建立5个员工的链表,并对链表进行创建、遍历、查找、和删除操作。
时间: 2023-12-18 21:05:26 浏览: 20
以下是在VS2013中编写的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Employee {
int ID;
float salary;
Employee* next;
};
// 创建员工链表
Employee* createEmployeeList() {
Employee* head = NULL;
Employee* tail = NULL;
for (int i = 0; i < 5; i++) {
Employee* emp = new Employee;
cout << "请输入第" << i+1 << "个员工的职工号:";
cin >> emp->ID;
cout << "请输入第" << i+1 << "个员工的工资:";
cin >> emp->salary;
emp->next = NULL;
if (head == NULL) {
head = emp;
tail = emp;
}
else {
tail->next = emp;
tail = emp;
}
}
return head;
}
// 遍历员工链表
void traverseEmployeeList(Employee* head) {
if (head == NULL) {
cout << "员工链表为空!" << endl;
return;
}
Employee* p = head;
while (p != NULL) {
cout << "职工号:" << p->ID << "\t工资:" << p->salary << endl;
p = p->next;
}
}
// 查找员工
Employee* findEmployee(Employee* head, int ID) {
if (head == NULL) {
cout << "员工链表为空!" << endl;
return NULL;
}
Employee* p = head;
while (p != NULL) {
if (p->ID == ID) {
return p;
}
p = p->next;
}
cout << "未找到职工号为" << ID << "的员工!" << endl;
return NULL;
}
// 删除员工
void deleteEmployee(Employee*& head, int ID) {
if (head == NULL) {
cout << "员工链表为空!" << endl;
return;
}
Employee* p = head;
Employee* pre = NULL;
while (p != NULL) {
if (p->ID == ID) {
if (pre == NULL) {
head = p->next;
delete p;
}
else {
pre->next = p->next;
delete p;
}
cout << "职工号为" << ID << "的员工已被删除!" << endl;
return;
}
pre = p;
p = p->next;
}
cout << "未找到职工号为" << ID << "的员工!" << endl;
}
int main() {
Employee* head = createEmployeeList();
cout << "创建员工链表完成!" << endl;
cout << "遍历员工链表:" << endl;
traverseEmployeeList(head);
int ID;
cout << "请输入要查找的员工的职工号:";
cin >> ID;
Employee* emp = findEmployee(head, ID);
if (emp != NULL) {
cout << "职工号为" << emp->ID << "的员工工资为:" << emp->salary << endl;
}
cout << "请输入要删除的员工的职工号:";
cin >> ID;
deleteEmployee(head, ID);
cout << "删除员工后的链表:" << endl;
traverseEmployeeList(head);
return 0;
}
```
在这个程序中,我们首先定义了一个员工结构体类型,包含职工号、工资和指针变量。然后,我们创建了一个函数 `createEmployeeList`,用于创建包含5个员工的链表。在创建链表时,我们通过动态内存分配 `new` 创建了每个员工结构体,并将他们连接成链表。接着,我们编写了函数 `traverseEmployeeList`,用于遍历员工链表并输出每个员工的职工号和工资。函数 `findEmployee` 用于查找指定职工号的员工并返回该员工的指针。最后,函数 `deleteEmployee` 用于删除指定职工号的员工。在 `main()` 函数中,我们先调用 `createEmployeeList` 函数创建员工链表,然后调用 `traverseEmployeeList` 函数遍历并输出链表中每个员工的信息。接着,我们让用户输入要查找的员工的职工号,并调用 `findEmployee` 函数查找该员工。如果该员工存在,则输出该员工的工资。接着,我们让用户输入要删除的员工的职工号,并调用 `deleteEmployee` 函数删除该员工。最后,我们再次调用 `traverseEmployeeList` 函数遍历并输出链表中每个员工的信息,以检查是否成功删除了指定员工。
相关推荐
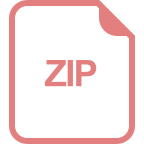







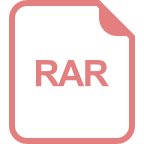
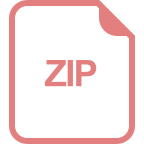
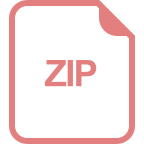
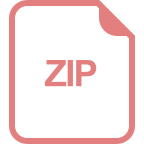
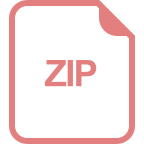
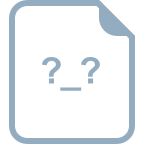