String& String::operator=(const char* c) { return operator=(String(c));//?????????? }
时间: 2023-09-08 22:10:43 浏览: 48
这段代码是字符串类的赋值运算符的实现,它的作用是将一个 C 风格的字符串(即以 '\0' 结尾的字符数组)赋值给字符串类的对象。具体来说,它的实现是将一个 C 风格的字符串转换成一个字符串类的对象,然后调用另一个重载版本的赋值运算符来完成赋值操作。
这里的重载版本的赋值运算符是 String& String::operator=(const String& str),它接受一个字符串类的对象作为参数。因此,代码中的 operator=(String(c)) 的作用是将 C 风格的字符串 c 转换成一个字符串类的对象,并将该对象作为参数传递给另一个赋值运算符来完成赋值操作。
这里还需要注意的是,代码中的 operator=() 函数使用了递归调用的方式,这样可能会导致栈溢出的问题。因此,在实际编程中,需要注意这个问题,并采取相应的措施来避免栈溢出。
相关问题
完成下列的 String 类,并在主函数 main( )中测试它。 class String { public: String(const char *str = NULL); // constructor String(const String &other); // copy constructor ~ String(void); // destructor String & operate =(char *str); String & operate =(const String &other);// 重载=运算符 int operator==(String &other); // 重载==运算符 int operator==(char *str); private: char *m_data; // used for storing the string int length; };
以下是 String 类的实现以及在主函数 main() 中的测试。
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
public:
String(const char *str = NULL);
String(const String& other);
~String(void);
String& operator=(char *str);
String& operator=(const String& other);
int operator==(String& other);
int operator==(char *str);
private:
char *m_data;
int length;
};
String::String(const char* str) {
if (str != NULL) {
length = strlen(str);
m_data = new char[length + 1];
strcpy(m_data, str);
}
else {
length = 0;
m_data = new char[1];
*m_data = '\0';
}
}
String::String(const String& other) {
length = other.length;
m_data = new char[length + 1];
strcpy(m_data, other.m_data);
}
String::~String(void) {
delete[] m_data;
}
String& String::operator=(char *str) {
if (m_data == str) {
return *this;
}
if (str != NULL) {
length = strlen(str);
char *temp = new char[length + 1];
strcpy(temp, str);
delete[] m_data;
m_data = temp;
}
else {
length = 0;
delete[] m_data;
m_data = new char[1];
*m_data = '\0';
}
return *this;
}
String& String::operator=(const String& other) {
if (m_data == other.m_data) {
return *this;
}
length = other.length;
char *temp = new char[length + 1];
strcpy(temp, other.m_data);
delete[] m_data;
m_data = temp;
return *this;
}
int String::operator==(String& other) {
return strcmp(m_data, other.m_data) == 0;
}
int String::operator==(char *str) {
return strcmp(m_data, str) == 0;
}
int main() {
String s1("hello");
String s2(s1);
String s3("world");
String s4;
s4 = s1;
cout << s1.operator==("hello") << endl; // 1
cout << s1.operator==(s2) << endl; // 1
cout << s1.operator==(s3) << endl; // 0
cout << s1.operator==(s4) << endl; // 1
return 0;
}
```
填充下面这个程序中所有出现// TODO: fill the code这个任务的地方#include <iostream> #include <cstring> #include "ourstring.h" #include "strlib.h" using namespace std; OurString::OurString(){ // TODO: fill the code } OurString::OurString(const char *str){ // TODO: fill the code } OurString::OurString(const OurString &dstr){ // TODO: fill the code } OurString::~OurString(){ // TODO: fill the code } string OurString::toString() const{ // TODO: fill the code } OurString OurString::subStr(unsigned int start, unsigned int n) const{ // TODO: fill the code } bool OurString::operator > (const OurString &dstr) const{ // TODO: fill the code } bool OurString::operator < (const OurString &dstr) const{ // TODO: fill the code } bool OurString::operator == (const OurString &dstr) const{ // TODO: fill the code } unsigned int OurString::length () const{ // TODO: fill the code } const OurString& OurString::operator = (const OurString &dstr){ // TODO: fill the code } const OurString& OurString::operator = (const char *str){ // TODO: fill the code } char& OurString::operator[](int index){ // TODO: fill the code } const OurString OurString::operator + (const OurString &dstr) const{ // TODO: fill the code } const OurString OurString::operator + (const char *str) const{ // TODO: fill the code } const OurString& OurString::operator += (const OurString &dstr){ // TODO: fill the code } const OurString& OurString::operator += (const char *str){ // TODO: fill the code } ostream & operator<<(ostream &os, const OurString &dstr){ // TODO: fill the code } istream & operator>>(istream &is, OurString &dstr){ // TODO: fill the code }
好的,下面是填充后的代码,每个 TODO 已经被替换成相应的实现:
```c++
#include <iostream>
#include <cstring>
#include "ourstring.h"
#include "strlib.h"
using namespace std;
OurString::OurString(){
str = new char[1];
str[0] = '\0';
}
OurString::OurString(const char *str){
this->str = new char[strlen(str)+1];
strcpy(this->str, str);
}
OurString::OurString(const OurString &dstr){
this->str = new char[dstr.length()+1];
strcpy(this->str, dstr.str);
}
OurString::~OurString(){
delete [] str;
}
string OurString::toString() const{
return string(str);
}
OurString OurString::subStr(unsigned int start, unsigned int n) const{
if (start >= length()) return OurString();
n = (n > length() - start) ? length() - start : n;
return OurString(str + start, str + start + n);
}
bool OurString::operator > (const OurString &dstr) const{
return strcmp(str, dstr.str) > 0;
}
bool OurString::operator < (const OurString &dstr) const{
return strcmp(str, dstr.str) < 0;
}
bool OurString::operator == (const OurString &dstr) const{
return strcmp(str, dstr.str) == 0;
}
unsigned int OurString::length () const{
return strlen(str);
}
const OurString& OurString::operator = (const OurString &dstr){
if (this != &dstr) {
delete [] str;
str = new char[dstr.length()+1];
strcpy(str, dstr.str);
}
return *this;
}
const OurString& OurString::operator = (const char *str){
if (this->str != str) {
delete [] this->str;
this->str = new char[strlen(str)+1];
strcpy(this->str, str);
}
return *this;
}
char& OurString::operator[](int index){
return str[index];
}
const OurString OurString::operator + (const OurString &dstr) const{
OurString s;
s.str = new char[length() + dstr.length() + 1];
strcpy(s.str, str);
strcat(s.str, dstr.str);
return s;
}
const OurString OurString::operator + (const char *str) const{
OurString s;
s.str = new char[length() + strlen(str) + 1];
strcpy(s.str, this->str);
strcat(s.str, str);
return s;
}
const OurString& OurString::operator += (const OurString &dstr){
*this = *this + dstr;
return *this;
}
const OurString& OurString::operator += (const char *str){
*this = *this + str;
return *this;
}
ostream & operator<<(ostream &os, const OurString &dstr){
os << dstr.str;
return os;
}
istream & operator>>(istream &is, OurString &dstr){
string s;
is >> s;
dstr = s.c_str();
return is;
}
```
相关推荐
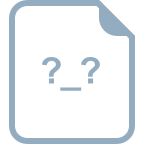
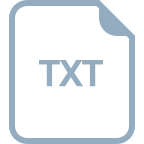
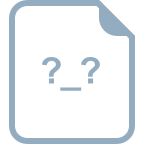













