使用C++语言实现一个集合(基于链表),需求如下:1.加入一个元素到集合中; 2.判断一个元素是否在集合中; 3.可以取两个集合的交集(重载“*”实现); 4.删除集合中的一个元素; 5.可以输出集合的所有元素(重载“<<”实现); 6.集合可以互相复制(可以永久保留集合内容)
时间: 2024-05-07 11:19:17 浏览: 7
以下是使用C语言实现一个基于链表的集合的代码。其中包含了加入元素、判断元素是否在集合中、取两个集合的交集、删除集合中的元素、输出集合的所有元素和集合互相复制的功能:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int value;
struct Node *next;
} Node;
typedef struct Set {
Node *head;
int size;
} Set;
// 创建一个新集合
Set* newSet() {
Set *set = (Set*) malloc(sizeof(Set));
set->head = NULL;
set->size = 0;
return set;
}
// 判断一个元素是否在集合中
int isElementInSet(Set *set, int value) {
Node *current = set->head;
while (current != NULL) {
if (current->value == value) {
return 1;
}
current = current->next;
}
return 0;
}
// 加入一个元素到集合中
void addToSet(Set *set, int value) {
if (!isElementInSet(set, value)) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->value = value;
newNode->next = set->head;
set->head = newNode;
set->size++;
}
}
// 删除集合中的一个元素
void removeFromSet(Set *set, int value) {
Node *current = set->head;
Node *previous = NULL;
while (current != NULL) {
if (current->value == value) {
if (previous == NULL) {
set->head = current->next;
} else {
previous->next = current->next;
}
free(current);
set->size--;
return;
}
previous = current;
current = current->next;
}
}
// 取两个集合的交集
Set* intersectionOfSets(Set *set1, Set *set2) {
Set *result = newSet();
Node *current = set1->head;
while (current != NULL) {
if (isElementInSet(set2, current->value)) {
addToSet(result, current->value);
}
current = current->next;
}
return result;
}
// 输出集合的所有元素
void printSet(Set *set) {
printf("{");
Node *current = set->head;
while (current != NULL) {
printf("%d", current->value);
if (current->next != NULL) {
printf(", ");
}
current = current->next;
}
printf("}\n");
}
// 集合互相复制
Set* copySet(Set *set) {
Set *result = newSet();
Node *current = set->head;
while (current != NULL) {
addToSet(result, current->value);
current = current->next;
}
return result;
}
int main() {
Set *set1 = newSet();
addToSet(set1, 1);
addToSet(set1, 2);
addToSet(set1, 3);
printf("Set 1: ");
printSet(set1);
Set *set2 = newSet();
addToSet(set2, 2);
addToSet(set2, 3);
addToSet(set2, 4);
printf("Set 2: ");
printSet(set2);
Set *intersection = intersectionOfSets(set1, set2);
printf("Intersection: ");
printSet(intersection);
removeFromSet(set1, 2);
printf("Set 1 after removing 2: ");
printSet(set1);
Set *copy = copySet(set1);
printf("Copy of set 1: ");
printSet(copy);
return 0;
}
```
该代码实现了一个基于链表的集合,包含了加入元素、判断元素是否在集合中、取两个集合的交集、删除集合中的元素、输出集合的所有元素和集合互相复制的功能。可以根据需要进行修改和扩展。
相关推荐
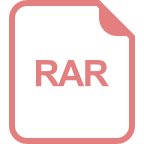














