实现论坛管理,1、在mysql中创建论坛表,包含留言时间、留言IP地址、留言内容,2、在Servlet程序中实现发表留言、留言显示、删除留言
时间: 2024-05-31 09:07:47 浏览: 24
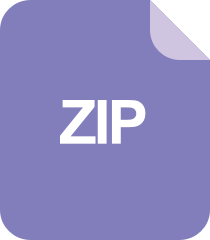
包含登陆,发布留言,获取IP和时间,可删除的php+mysql留言簿

1. 在MySQL中创建论坛表
CREATE TABLE forum (
id INT NOT NULL AUTO_INCREMENT PRIMARY KEY,
time TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
ip VARCHAR(45) NOT NULL,
content TEXT NOT NULL
);
2. 在Servlet程序中实现发表留言、留言显示、删除留言
发表留言:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String ip = request.getRemoteAddr();
String content = request.getParameter("content");
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement stmt = conn.prepareStatement("INSERT INTO forum (ip, content) VALUES (?, ?)");
stmt.setString(1, ip);
stmt.setString(2, content);
stmt.executeUpdate();
stmt.close();
conn.close();
response.sendRedirect("forum.jsp");
} catch (SQLException e) {
e.printStackTrace();
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
}
}
留言显示:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM forum ORDER BY time DESC");
List<Message> messages = new ArrayList<Message>();
while (rs.next()) {
int id = rs.getInt("id");
Timestamp time = rs.getTimestamp("time");
String ip = rs.getString("ip");
String content = rs.getString("content");
Message message = new Message(id, time, ip, content);
messages.add(message);
}
rs.close();
stmt.close();
conn.close();
request.setAttribute("messages", messages);
request.getRequestDispatcher("forum.jsp").forward(request, response);
} catch (SQLException e) {
e.printStackTrace();
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
}
}
删除留言:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement stmt = conn.prepareStatement("DELETE FROM forum WHERE id = ?");
stmt.setInt(1, id);
stmt.executeUpdate();
stmt.close();
conn.close();
response.sendRedirect("forum.jsp");
} catch (SQLException e) {
e.printStackTrace();
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
}
}
阅读全文
相关推荐
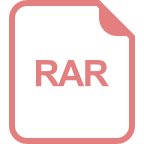
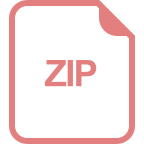


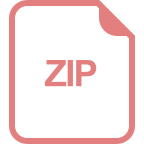
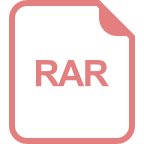
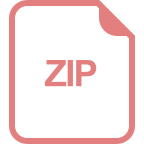
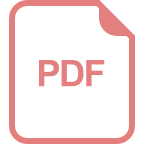
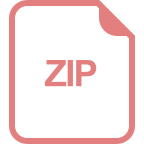
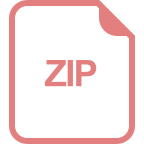
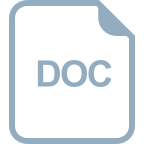
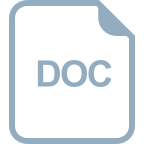
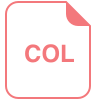
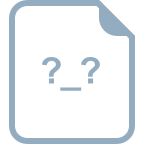
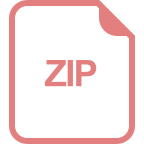