上体育课的时候,老师已经把班级同学排成了两个队列,而且每个队列都是按照从底到高排好队,现在需要把两个队列合并,合并后需要保证还是从低到高排列。合并队列,你能用C++编程实现吗
时间: 2024-01-10 20:05:08 浏览: 22
当然可以,下面是C++的代码实现:
```cpp
#include <iostream>
#include <queue>
using namespace std;
// 合并两个有序队列
queue<int> merge(queue<int> q1, queue<int> q2)
{
queue<int> q;
while (!q1.empty() && !q2.empty())
{
if (q1.front() <= q2.front())
{
q.push(q1.front());
q1.pop();
}
else
{
q.push(q2.front());
q2.pop();
}
}
while (!q1.empty())
{
q.push(q1.front());
q1.pop();
}
while (!q2.empty())
{
q.push(q2.front());
q2.pop();
}
return q;
}
int main()
{
// 测试数据
queue<int> q1, q2;
q1.push(1);
q1.push(3);
q1.push(5);
q2.push(2);
q2.push(4);
q2.push(6);
// 合并两个队列
queue<int> q = merge(q1, q2);
// 输出合并后的序列
while (!q.empty())
{
cout << q.front() << " ";
q.pop();
}
cout << endl;
return 0;
}
```
以上代码中,我们定义了一个`merge`函数,它接收两个有序队列作为参数,返回一个合并后的有序队列。在函数实现中,我们使用了两个while循环,分别将两个队列中的元素逐一取出比较,然后放入新的队列中,最终返回合并后的队列。
在主函数中,我们定义了两个测试数据的队列,分别为`q1`和`q2`,然后调用`merge`函数将它们合并成一个新的队列`q`,最后输出合并后的序列。
相关推荐
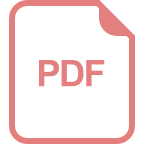



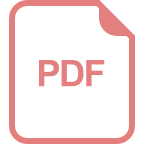
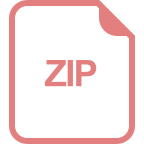
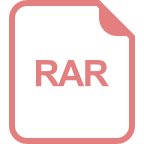
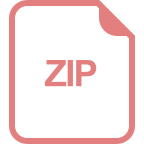
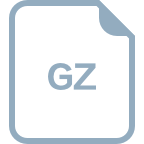
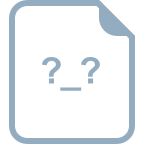
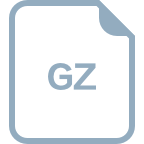