how to convert a docx file to html file use docx4j
时间: 2024-04-08 07:28:48 浏览: 121
To convert a DOCX file to an HTML file using docx4j, you can follow these steps:
1. Add the docx4j dependency to your project. You can do this by including the following Maven dependency in your project's pom.xml file:
```xml
<dependency>
<groupId>org.docx4j</groupId>
<artifactId>docx4j</artifactId>
<version>8.2.10</version>
</dependency>
```
2. Load the DOCX file using docx4j. Here's an example:
```java
import org.docx4j.Docx4J;
import org.docx4j.convert.out.HTMLSettings;
import org.docx4j.openpackaging.packages.WordprocessingMLPackage;
public class DocxToHtmlConverter {
public static void main(String[] args) throws Exception {
// Load the DOCX file
WordprocessingMLPackage wordMLPackage = Docx4J.load(new File("input.docx"));
// Setup HTML conversion options
HTMLSettings htmlSettings = Docx4J.createHTMLSettings();
// Convert the DOCX to HTML
String htmlOutput = Docx4J.toHTML(htmlSettings, wordMLPackage.getMainDocumentPart());
// Save the HTML output to a file
FileUtils.writeStringToFile(new File("output.html"), htmlOutput, "UTF-8");
}
}
```
Make sure to replace "input.docx" with the path to your actual input DOCX file and "output.html" with the desired output HTML file path.
3. Run the code, and it will generate the HTML output file.
Note that docx4j may not support all features of complex DOCX files, so the resulting HTML might not be an exact representation of the original document. You may need to adjust the generated HTML or use additional libraries to achieve the desired output.
阅读全文
相关推荐




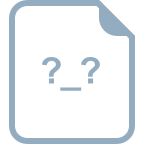
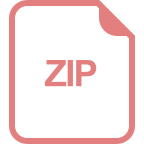
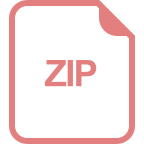
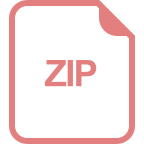
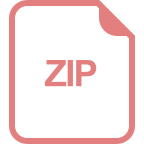
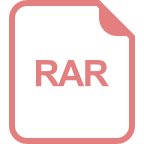
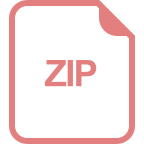