用java语言写UsersDao类,该类主要用于程序与数据库的交互,在该类中封装了对数据库表users的添加、查询、删除和更新等操作。
时间: 2023-06-13 15:07:35 浏览: 43
下面是一个Java语言的UsersDao类的示例代码,该类主要用于与数据库表users进行交互,并封装了添加、查询、删除和更新等操作:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UsersDao {
// 数据库连接相关信息
private String driver = "com.mysql.jdbc.Driver";
private String url = "jdbc:mysql://localhost:3306/test";
private String username = "root";
private String password = "root";
// 添加用户
public boolean addUser(User user) {
Connection conn = null;
PreparedStatement pstmt = null;
int result = 0;
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, username, password);
String sql = "insert into users(username, password, email) values(?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getEmail());
result = pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return result > 0;
}
// 删除用户
public boolean deleteUser(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
int result = 0;
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, username, password);
String sql = "delete from users where id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
result = pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return result > 0;
}
// 更新用户
public boolean updateUser(User user) {
Connection conn = null;
PreparedStatement pstmt = null;
int result = 0;
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, username, password);
String sql = "update users set username=?, password=?, email=? where id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getEmail());
pstmt.setInt(4, user.getId());
result = pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return result > 0;
}
// 查询用户
public User getUser(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
User user = null;
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, username, password);
String sql = "select * from users where id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
rs = pstmt.executeQuery();
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setEmail(rs.getString("email"));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return user;
}
}
```
在以上代码中,我们使用了JDBC进行数据库连接,并封装了添加、查询、删除和更新等操作。其中,我们使用了预处理语句PreparedStatement进行操作,这样可以有效地防止SQL注入攻击。同时,在每个方法中,我们都进行了try-catch-finally的异常处理和资源释放操作。
阅读全文
相关推荐


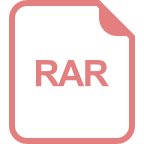

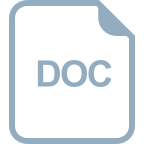









import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.sql.SQLException; @WebServlet("/update") public class update extends HttpServlet { @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { resp.setContentType("text/html;charset=utf-8"); String id = req.getParameter("id"); String username = req.getParameter("username"); String password = req.getParameter("password"); UsersDao usersDao = new UsersDao(); User user = new User(); user.setUsername(username); user.setPassword(password); user.setId(Integer.valueOf(id)); try { if (usersDao.update(user)) { resp.getWriter().println("修改成功"+"返回主页"); } else { resp.getWriter().println("修改失败"+"返回主页"); } } catch (SQLException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } } }

