帮我编写一个python程序,实现画一个圣诞树
时间: 2023-09-11 11:05:20 浏览: 126
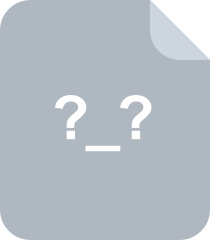
用Python画一幅圣诞树
### 回答1:
好的,为了帮助你画出一棵圣诞树,我们可以使用 Python 的绘图库 matplotlib 来实现。
首先,我们需要安装 matplotlib,可以使用以下命令安装:
```
pip install matplotlib
```
然后,我们就可以开始编写代码了。
首先,我们需要导入 matplotlib 的 pyplot 库,并使用其中的 triangle 函数来画出一个三角形,这就是我们的圣诞树的基础。
```python
import matplotlib.pyplot as plt
# 绘制三角形,填充绿色
plt.fill([0, 0, 1], [0, 1, 0], color='green')
plt.show()
```
接下来,我们可以使用 fill 函数来填充圣诞树的圆圈,并添加一些圣诞装饰。
```python
import matplotlib.pyplot as plt
# 绘制三角形,填充绿色
plt.fill([0, 0, 1], [0, 1, 0], color='green')
# 绘制圆圈,填充红色
plt.fill([0.25, 0.5, 0.75], [0.5, 0.75, 0.5], color='red')
# 绘制五角星,填充黄色
plt.fill([0.5, 0.61, 0.68, 0.5, 0.32, 0.39], [0.75, 0.83, 0.92, 0.96, 0.92, 0.83], color='yellow')
plt.show()
```
希望这些代码能帮助你实现你想要的圣诞树。
### 回答2:
下面是一个用 Python 编写的画圣诞树的程序:
```python
def draw_christmas_tree(height):
for i in range(1, height+1):
print(' '*(height-i) + '*'*(2*i-1))
print(' '*(height-1) + '|')
height = int(input("请输入圣诞树的高度:"))
draw_christmas_tree(height)
```
这个程序实现了一个 `draw_christmas_tree` 函数,通过传入 `height` 参数来确定圣诞树的高度。每一行的字符数是通过 `2*i-1` 计算得到的,其中 `i` 表示当前行数。最后一行是树干,只有一个字符 `|`。
### 回答3:
当然,我很乐意帮你编写一个Python程序,实现画一个圣诞树!你可以尝试以下的代码:
```python
def draw_christmas_tree(height):
for i in range(height):
print(" " * (height - i - 1) + "*" * (2 * i + 1))
print(" " * (height - 1) + "|")
height = int(input("请输入圣诞树的高度:"))
draw_christmas_tree(height)
```
这段代码首先定义了一个名为`draw_christmas_tree`的函数,它接收一个参数`height`作为圣诞树的高度。然后,使用一个循环来绘制每一层的圣诞树,通过在每一层分别打印空格和星号来形成三角形状的圣诞树。最后,在最底部打印一根竖线作为树干。
最后,主程序部分会提示用户输入圣诞树的高度,并将用户输入的高度作为参数传递给`draw_christmas_tree`函数,从而绘制出指定高度的圣诞树。
希望这个代码能满足你的需求,祝你圣诞节快乐!
阅读全文
相关推荐


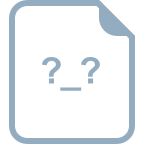
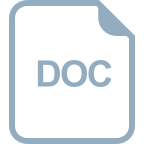
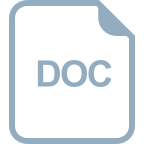
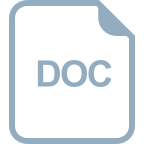
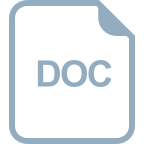







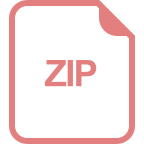
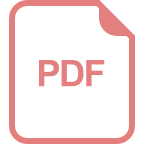