爬取给定网址中的文本信息,并存储到doc格式文件中,增加头文件反爬,写成函数,直接写python代码
时间: 2023-09-08 17:04:13 浏览: 106
### 回答1:
好的,我会尽力帮你。
首先,我们需要使用 Python 的第三方库 requests 来发送 HTTP 请求,并使用 beautifulsoup4 来解析网页。我们还需要使用 python-docx 库来创建 doc 文件。
安装这些库的方法是:
```
pip install requests beautifulsoup4 python-docx
```
然后,我们可以使用下面的代码来爬取网页并将其存储在 doc 文件中:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_save(url, headers, doc_name):
# 发送 HTTP 请求
response = requests.get(url, headers=headers)
# 解析网页
soup = BeautifulSoup(response.text, "html.parser")
# 获取文本信息
text = soup.get_text()
# 创建 doc 文件
document = Document()
document.add_paragraph(text)
# 保存文件
document.save(doc_name)
# 调用函数
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/87.0.4280.88 Safari/537.36"
}
crawl_and_save("https://www.example.com", headers, "example.docx")
```
这段代码会发送一个带有自定义头部的 HTTP 请求到给定的 URL,并使用 beautifulsoup4 库解析网页。然后,它会使用 get_text() 方法获取网页的文本信息,并使用 python-docx 库创建一个 doc 文件,将文本添加到文件中,最后保存文件。
希望这些内容对你有帮助。
### 回答2:
可以使用Python的requests库和BeautifulSoup库实现爬取给定网址中的文本信息,并存储到doc格式文件中。为了防止被反爬虫,我们可以为爬虫程序增加头文件。
以下是一个实现该功能的示例代码:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_save(url, filename):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
# 通过选择器获取需要爬取的文本信息
text_elements = soup.select('p') # 这里使用<p>标签作为示例
doc = Document()
for element in text_elements:
text = element.get_text()
doc.add_paragraph(text)
doc.save(filename)
# 测试
url = 'https://example.com' # 替换为要爬取的网址
filename = 'output.docx' # 保存的文件名
crawl_and_save(url, filename)
```
请将代码中的`url`和`filename`变量替换为实际的网址和保存的文件名。在代码中,我们首先定义了一个`headers`字典,其中包含了请求的头文件信息。然后使用`requests.get`方法发送HTTP请求,并传入`headers`参数。接着使用BeautifulSoup库解析返回的HTML响应,并用选择器获取需要爬取的文本信息。最后,将获取到的文本信息添加到一个docx文档中,并保存为指定的文件。
### 回答3:
爬取给定网址中的文本信息并存储到doc格式文件中可以使用Python的requests库和python-docx库来实现。以下是一个具体的示例代码:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_store(url):
# 添加头文件,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
# 发送GET请求获取网页内容
response = requests.get(url, headers=headers)
response.encoding = 'utf-8' # 设置文本编码
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 提取需要的文本信息
text = soup.get_text()
# 创建Word文档对象
doc = Document()
doc.add_paragraph(text) # 将文本信息添加到文档
# 保存文档为doc格式
doc.save('text.doc')
# 测试
url = 'http://example.com' # 替换为需要的网址
crawl_and_store(url)
```
上述代码中,首先使用requests库发送GET请求获取网页内容,并加入了模拟浏览器访问的头文件,以防止反爬机制的限制。然后使用BeautifulSoup库解析网页内容,提取所需的文本信息。接下来,使用python-docx库创建一个Word文档对象,并将文本信息添加到文档中。最后,将文档保存为doc格式文件。
阅读全文
相关推荐















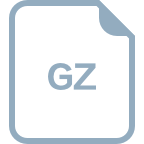