qt opencv摄像头
时间: 2023-06-13 15:06:58 浏览: 94
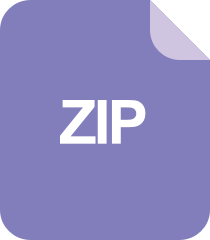
qt与cv调用电脑的摄像头练习
在 Qt 中使用 OpenCV 库来获取摄像头视频流的步骤如下:
1. 首先,你需要在 Qt 项目中添加 OpenCV 库。你可以在 Qt 项目的 .pro 文件中添加以下行:
```
INCLUDEPATH += /path/to/opencv/include
LIBS += -L/path/to/opencv/lib -lopencv_core -lopencv_highgui -lopencv_videoio
```
请确保修改 `/path/to/opencv` 为你的 OpenCV 安装路径。
2. 在 Qt 的界面中添加一个 QLabel 控件来显示摄像头视频流。
3. 创建一个 OpenCV 的 VideoCapture 对象,打开摄像头。
```c++
#include <opencv2/videoio.hpp>
cv::VideoCapture cap(0); // 打开默认摄像头(编号为 0)
if (!cap.isOpened()) {
// 打开摄像头失败
}
```
4. 在一个循环中不断读取摄像头的帧,并将其显示在 QLabel 控件中。
```c++
while (true) {
cv::Mat frame;
if (!cap.read(frame)) {
// 读取帧失败
break;
}
// 将帧转换为 QImage,以便在 QLabel 中显示
QImage qimage(frame.data, frame.cols, frame.rows, frame.step, QImage::Format_RGB888);
ui->label->setPixmap(QPixmap::fromImage(qimage));
ui->label->repaint(); // 强制刷新 QLabel 控件
QCoreApplication::processEvents(); // 处理界面事件
}
```
请注意,在循环中使用 `QCoreApplication::processEvents()` 处理界面事件是必要的,这样才能保证界面响应正常。
完整的示例代码如下:
```c++
#include <opencv2/videoio.hpp>
#include <QCoreApplication>
#include <QImage>
#include <QLabel>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建 OpenCV 的 VideoCapture 对象,打开摄像头
cv::VideoCapture cap(0); // 打开默认摄像头(编号为 0)
if (!cap.isOpened()) {
// 打开摄像头失败
return -1;
}
// 在 Qt 的界面中添加一个 QLabel 控件来显示摄像头视频流
QLabel *label = new QLabel();
label->show();
// 不断读取摄像头的帧,并将其显示在 QLabel 控件中
while (true) {
cv::Mat frame;
if (!cap.read(frame)) {
// 读取帧失败
break;
}
// 将帧转换为 QImage,以便在 QLabel 中显示
QImage qimage(frame.data, frame.cols, frame.rows, frame.step, QImage::Format_RGB888);
label->setPixmap(QPixmap::fromImage(qimage));
label->repaint(); // 强制刷新 QLabel 控件
QCoreApplication::processEvents(); // 处理界面事件
}
return a.exec();
}
```
希望对你有所帮助!
阅读全文
相关推荐
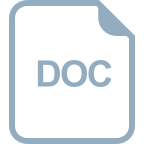
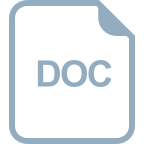
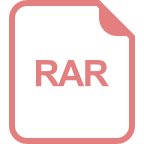


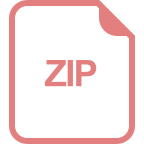
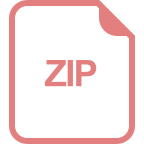

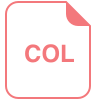





