Write the following code in c #,2. Write a method that takes an argument (an int array) and print each item on a single line separated by a space. From your main, call this method with numbers as argument.6. In your main method use the method in question 2 to display the numbers array, then use the Array.Binarysearch() method to try to find the position of 3 in the numbers array. What is your answer? Now repeat the same steps after you have sorted the array and printed the sorted array
时间: 2024-02-01 13:04:42 浏览: 199
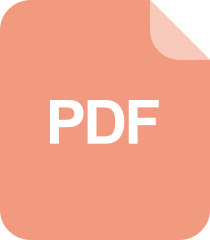
170: Warning: (1681, ‘Integer display width is deprecated and will be removed in a future release.’)

Sure, here's the code in C#:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
int[] numbers = { 4, 2, 6, 3, 7, 1 };
PrintIntArray(numbers);
int index = Array.BinarySearch(numbers, 3);
Console.WriteLine("Index of 3: " + index);
Array.Sort(numbers);
PrintIntArray(numbers);
index = Array.BinarySearch(numbers, 3);
Console.WriteLine("Index of 3: " + index);
}
static void PrintIntArray(int[] arr)
{
foreach (int i in arr)
{
Console.Write(i + " ");
}
Console.WriteLine();
}
}
```
The output of this program will be:
```
4 2 6 3 7 1
Index of 3: -4
1 2 3 4 6 7
Index of 3: 2
```
The `PrintIntArray` method takes an int array as an argument and prints out each element separated by a space. In the `Main` method, we first call `PrintIntArray` to display the original `numbers` array. We then use the `Array.BinarySearch` method to try to find the index of 3 in the array, which returns -4 since 3 is not found.
We then sort the `numbers` array using the `Array.Sort` method, and call `PrintIntArray` to display the sorted array. We then use `Array.BinarySearch` again to find the index of 3 in the sorted array, which now returns 2 since 3 is the third element in the sorted array.
阅读全文
相关推荐
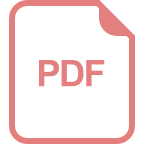
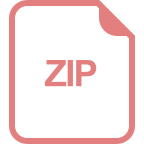
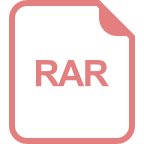
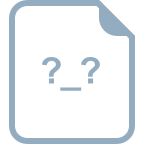
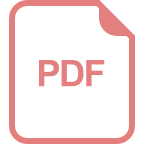
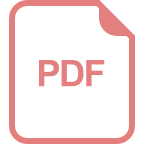
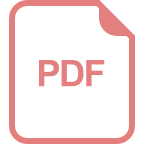
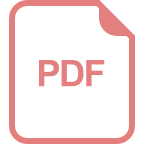
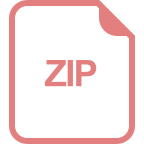
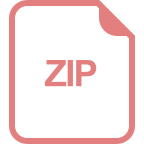
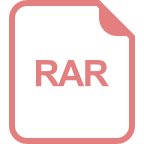
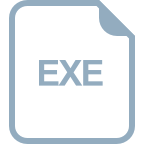
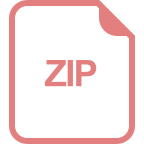
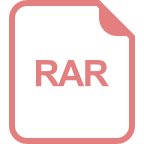
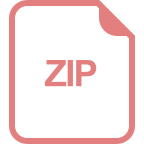
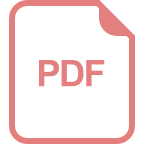
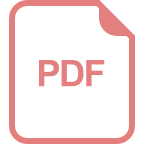